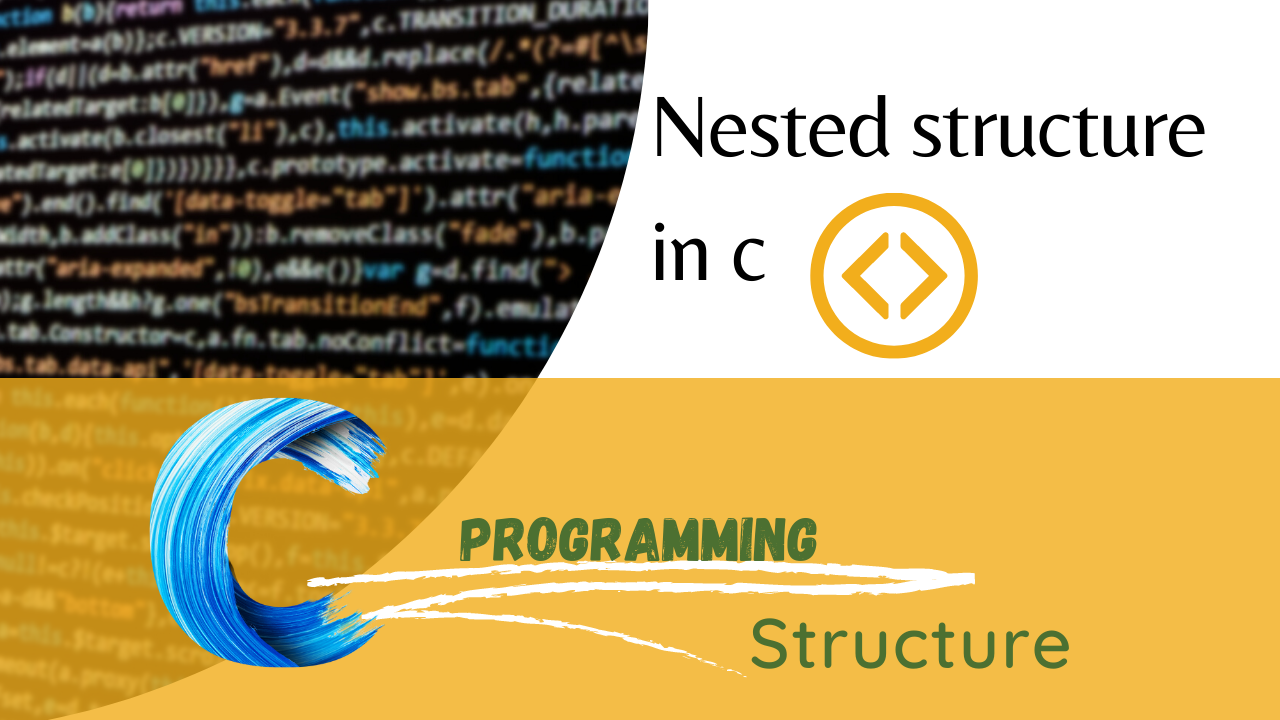
Nested structure in c
Mastering Nested Structures in C for Advanced Programming
A nested structure in C is a structure that contains one or more members that are themselves structures. This allows for the creation of complex data structures that can be used to represent real-world objects.
For example, consider the following nested structure:
struct Employee {
char name[30];
int age;
struct Address {
char street[50];
char city[50];
char state[50];
int zipCode;
} address;
};
This nested structure can be used to represent an employee, including their name, age, and address. The address member of the Employee structure is itself a structure, which allows us to store the employee's address in a single variable.
Nested structures can be nested to any depth. This means that a structure can contain a member that is another structure, which can in turn contain a member that is another structure, and so on.
Nested structures can be useful for organizing complex data in a way that is easy to understand and maintain. They can also be used to improve the performance of programs by reducing the amount of code that needs to be written.
Some examples of how nested structures can be used:
- To represent a hierarchical data structure, such as a file system or a tree.
- To represent complex objects, such as a student record or a customer order.
- To store data that is related to each other, such as an employee's name, address, and phone number.
Example of how to use the Nested structure:
struct Employee employee;
strcpy(employee.name, "John Doe");
employee.age = 30;
strcpy(employee.address.street, "123 Main Street");
strcpy(employee.address.city, "Anytown");
strcpy(employee.address.state, "CA");
employee.address.zipCode = 91234;
printf("Employee name: %s\n", employee.name);
printf("Employee age: %d\n", employee.age);
printf("Employee address: %s, %s, %s, %d\n", employee.address.street, employee.address.city, employee.address.state, employee.address.zipCode);
Output:
Employee name: John Doe
Employee age: 30
Employee address: 123 Main Street, Anytown, CA, 91234
Top Resources
what is structure in C?
What is self-referential structure pointer in c?
What is structure padding? How to avoid structure padding?
differences between a structure and a union in c?
How can typedef be to define a type of structure in c?
Program to illustrate a function that assigns value to the structure
What is structure? What are differences between Arrays and Structures?
Write a program to find the sizeof structure without using sizeof operator?
Write a program that returns 3 numbers from a function using a structure in c.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!
5:20:2021