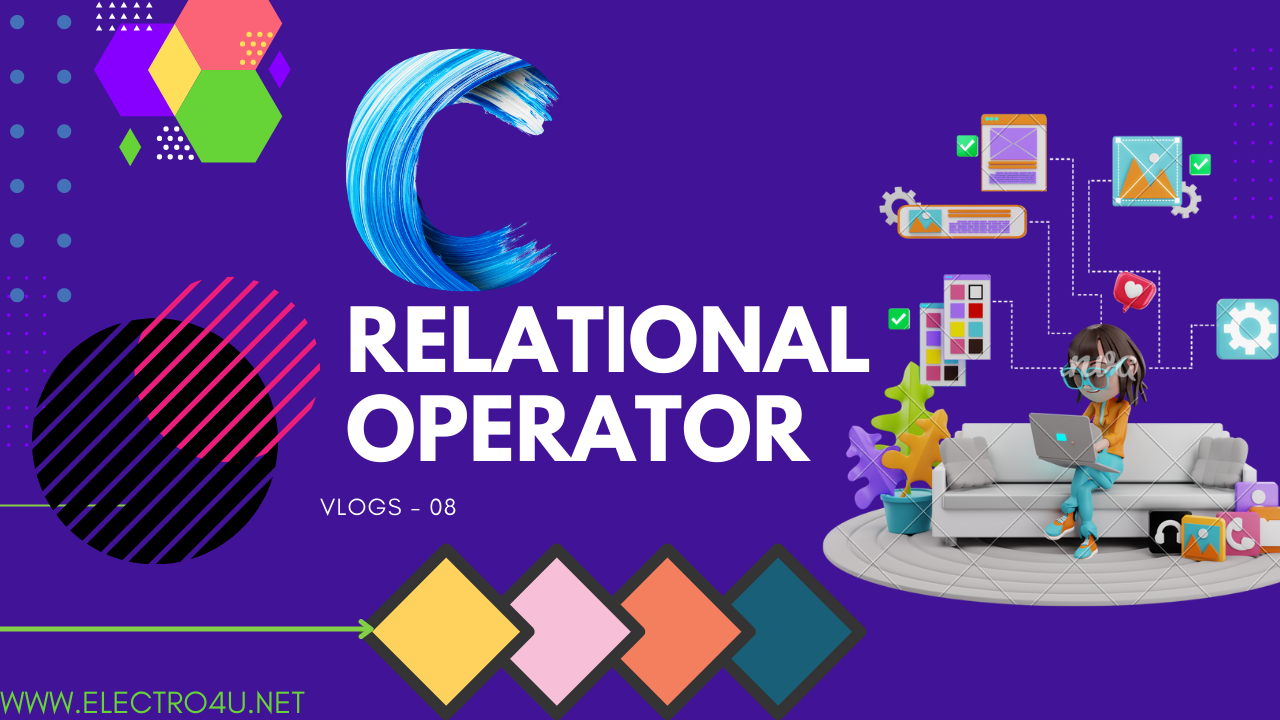
Relational operator in c programming
Relational Operators in C Programming
Relational operators are used to compare the values of two expressions depending on their relations. An expression that contains relational operators is called a relational expression. If the relation is true then the value of the relational expression is 1 and if the relation is false then the value of the expression is 0.
List of relational operators
Operator |
|
> | Greater than |
>= | Greater than equal to |
< | less then |
<= | Less than equal to |
== | equal to |
!= | Not equal to |
Notes:
- All these Relational Operators are binary Operators because it's required two Operands
- All these Operator produce the result 0 or 1 (true or false)
- These operators will not modify the operand, so we can supply variables as well as constant
Example: Let us take two variables i=20 and b=10,
expression | Relation | Value of expression |
i<=j | False | 0 |
i==j | False | 0 |
i!=j | True | 1 |
i>8 | True | 1 |
i!=0 | True | 1 |
i>j | True | 1 |
Conclusion: It is important to note that the assignment operator(=) and equality operator(==) are entirely different. The assignment operator is used for assigning values while the equality operator is used to compare two expressions. Beginners generally confuse the two and use one in place of another, and this leads to an error difficult to find out.
see on program 01
Program 01: C program to demnstrate Relational Operators
#include
main()
{
int i=10,j=20;
printf("%d\n",i==j);
printf("%d\n",i=j);
}
Output: 0 20
Solution: The first print statement will compare i and j variables and will give the output as zero(0) as they are not the same. The second print statement will assign the value of j to variable i and will give the output as 20.
Program 02: Write c Program to understand Relational Operation greater then operation
#include
void main()
{
int i=1,j=20,k;
k=i>j;
printf("k=%d\n",k);
}
Output: 0
Attention: In this Program, we compare the value i Greater than j or not if not then the given condition is Flase is if greater the given condition is Correct but In this program the given condition is incorrect That's why we got output is ZERO
Program 03: Program to understand the use of relational operators
#include
int main(void)
{
int a,b ;
printf("Enter values for a and b : ");
scanf("%d%d",&a,&b);
if(a<b)
printf("%d is less than %d\n",a,b);
if(a<=b)
printf("%d is less than or equal to %d\n",a,b);
if(a==b)
printf("%d is equal to %d\n",a,b);
if(a!=b)
printf("%d is not equal to %d\n",a,b);
if(a>b)
printf("%d is greater than %d\n",a,b);
if(a>=b)
printf("%d is greater than or equal to %d\n",a,b);
return 0;
}
Solution: The program first asks the user to enter two integers, a and b. Then, the program uses a series of if statements to compare the two integers and print out the appropriate comparison. For example, if a is less than b, the program will print out %d is less than %d.
The following is a breakdown of the program:
- #include: This line tells the compiler to include the stdio.h header file, which contains declarations for the printf() and scanf() functions.
- int main(void): This line defines the main() function, which is the entry point for all C programs.
- int a,b ;: This line declares two integer variables, a and b.
- printf("Enter values for a and b : ");: This line prints a prompt to the user asking them to enter two integers.
- scanf("%d%d",&a,&b);: This line reads two integers from the user and stores them in the a and b variables.
- if(a<b) printf("%d is less than %d\n",a,b);: This line uses an if statement to compare the a and b variables. If a is less than b, the program will print out %d is less than %d.
- if(a<=b) printf("%d is less than or equal to %d\n",a,b);: This line uses an if statement to compare the a and b variables. If a is less than or equal to b, the program will print out %d is less than or equal to %d.
- if(a==b) printf("%d is equal to %d\n",a,b);: This line uses an if statement to compare the a and b variables. If a is equal to b, the program will print out %d is equal to %d.
- if(a!=b) printf("%d is not equal to %d\n",a,b);: This line uses an if statement to compare the a and b variables. If a is not equal to b, the program will print out %d is not equal to %d.
- if(a>b) printf("%d is greater than %d\n",a,b);: This line uses an if statement to compare the a and b variables. If a is greater than b, the program will print out %d is greater than %d.
- if(a>=b) printf("%d is greater than or equal to %d\n",a,b);: This line uses an if statement to compare the a and b variables. If a is greater than or equal to b, the program will print out %d is greater than or equal to %d.
- return 0;: This line returns the value 0 from the main() function, indicating that the program has terminated successfully.