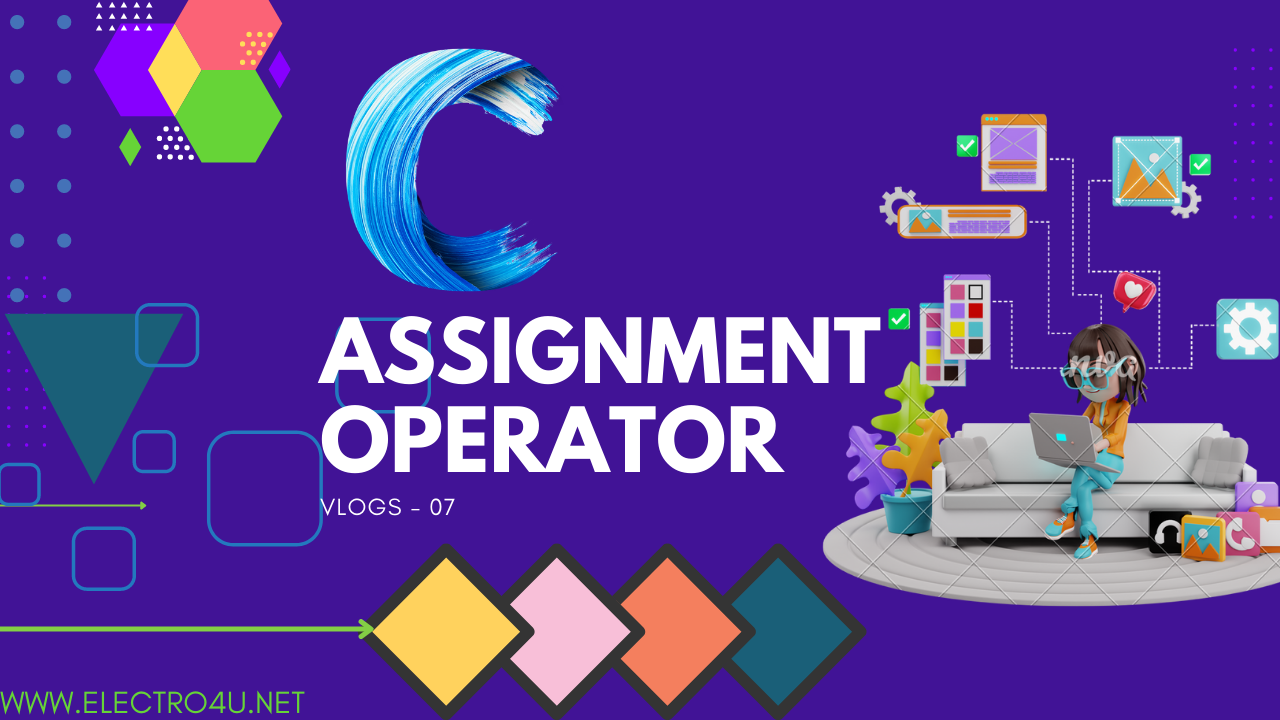
Assignment operator in c programming
Assignment Operator in C Programming
The assignment operator in C programming is used to assign a value to a variable. The assignment operator is represented by the equal sign (=).
To assign a value to a variable, we simply place the value to the right of the assignment operator and the variable name to the left of the assignment operator. For example, the following code assigns the value 10 to the variable x:
int x = 10;
The assignment operator can also be used to assign the value of one variable to another variable. For example, the following code assigns the value of the variable x
to the variable y:
int x = 10;
int y = x;
The assignment operator can also be used to assign the result of an expression to a variable. For example, the following code assigns the result of the expression 10 + 20
to the variable z
:
int z = 10 + 20;
The assignment operator is one of the most important operators in C programming. It is used to assign values to variables, which is essential for writing any C program.
Examples of how to use the assignment operator in C programming:
// Assign the value 10 to the variable x.
int x = 10;
// Assign the value of the variable x to the variable y.
int y = x;
// Assign the result of the expression 10 + 20 to the variable z.
int z = 10 + 20;
// Assign the value of the variable a to the array element arr[0].
int arr[10];
arr[0] = a;
// Assign the value of the array element arr[0] to the variable b.
int b = arr[0];
The assignment operator is a powerful tool that can be used to write efficient and concise C code.
Program 01:
#include<stdio.h>
int main()
{
int i=10,j;
j=i;//valid expression expression
//10=j; invalid expression
printf("i=%d j=%d\n",i,j);
}
conclusion:
The meaning of Assignment Operator is first to perform the operator that we give then assing the value in the left-hand side Operand
Top Resources
Arithmetic operator in c
Assignment operator in c programming
Relational operator in c programming
Logical Operator in c programming
Bitwise operator in c programming
sizeof operator in c programming
Understanding the Comma Operator in C Programming
Dot Operator in c programming
Using && Logical AND Operator in C Programming
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!