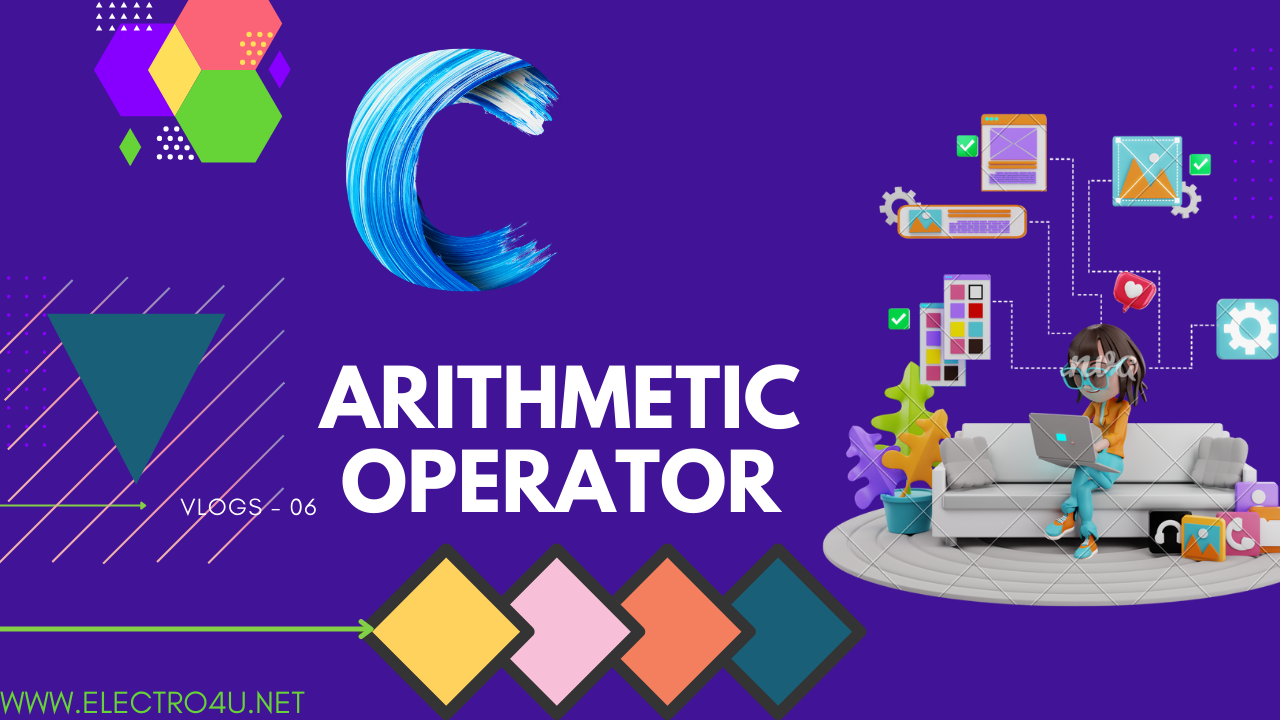
Arithmetic operator in c
C Programming Tutorial: Basic Arithmetic Operators
Arithmetic operator: + - * / %
Arithmetic operators are used for numeric calculations. all the Arithematic operators are binary operators which required two operands to perform the task. all these arithmetic operators will not modify the operand
C is a programming language that includes a variety of arithmetic operators. These operators allow you to perform basic arithmetic operations on numerical values.
Here are the arithmetic operators available in C:
-
Addition (+): The addition operator adds two values together.
-
Subtraction (-): The subtraction operator subtracts one value from another.
-
Multiplication (*): The multiplication operator multiplies two values together.
-
Division (/): The division operator divides one value by another.
-
Modulus (%): The modulus operator returns the remainder of a division operation.
Example: here i and j are two operands
i=10, j=20,k=i+j;
k=i+j;
output: k=30
operators are works based on associativity and priority. if we have to encounter the same type of priority-based operator at the same time that time solves expression as per the associativity of an operand.
Note 01:
' / '
it will give the coefficient
' % '
it will give a reminder
Example:
13/12= 6 ( 6 is the coefficient)
13%12=1 (1 is a reminder)
Note 01: Basic function of Divided by 10 or modules by 10
123%10 = extract last digit(means reminder)
123/10= delete last digit
Example 02
123%10= 3( we get)
123/10= 12 we get
⇒Modulus operator cannot be applicable to real numbers. if we apply then we got a syntactical error
Real number
- Float
- double
Program 01: swapping of two numbers using the arithmetic operator (+ -)
#include<stdio.h>
int main()
{
int n1,n2;
printf("Enter two number n1 & n2:");
scanf("%d%d",&n1,&n2);
printf("Before swapping n1:%d and n2:%d\n",n1,n2);
n1=n1+n2;
n2=n1-n2;
n1=n1-n2;
printf("After swapping n1:%d and n2:%d\n",n1,n2);
}
output:
Enter two numbers n1 & n2:10 20
Before swapping n1:10 and n2:20
After swapping n1:20 and n2:10
Program 02: Swapping of two numbers using Multiplication and division operator
#include<stdio.h>
int main()
{
int n1,n2;
printf("Enter two number n1 & n2:");
scanf("%d%d",&n1,&n2);
printf("Before swapping n1:%d and n2:%d\n",n1,n2);
n1=n1*n2;
n2=n1/n2;
n1=n1/n2;
printf("After swapping n1:%d and n2:%d\n",n1,n2);
}
Output:
Enter two number n1 & n2:50 60
Before swapping n1:50 and n2:60
After swapping n1:60 and n2:50