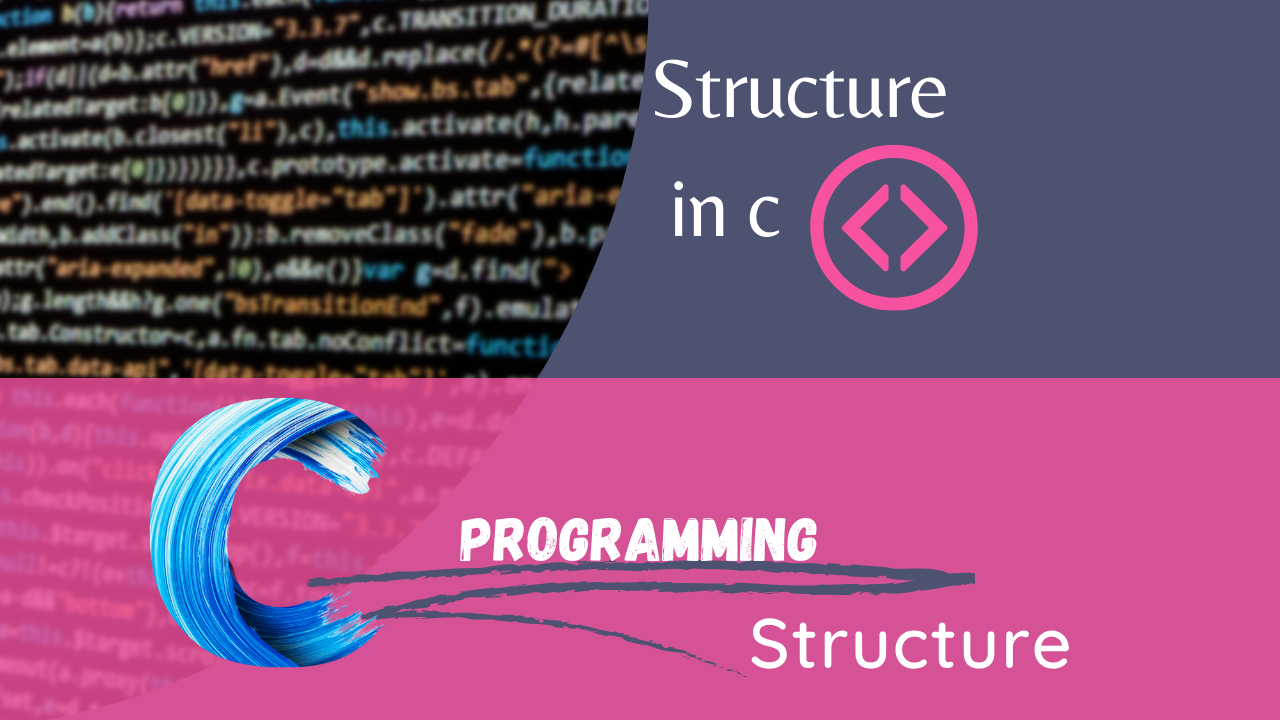
Structure in c
Understanding Structures in C
Introduction
Structures are a fundamental concept in the C programming language. They allow you to group together variables of different types under a single name. This makes it easier to organize and manage complex data in your programs.
Before starting this chapter views things to know
User-defined Data type:
- Structure
- union
- Enum
- typedef
What is a Structure?
A structure in C is a user-defined data type that allows you to combine different types of variables under a single name. Each variable within a structure is called a member or field. These members can be of any data type, including basic types like integers and floats, or even other structures.
Declaring a Structure
To define a structure, you use the struct keyword followed by the name you choose for your structure. For example:
struct Person {
char name[50];
int age;
float height;
};
In this example, we've defined a structure called Person with three members: name, age, and height.
Accessing Members
You can access the members of a structure using the dot (.) operator. For example:
Note: When we initialization structure variable order is very important
Few rules and regulations we need to follow when we are using the structure
- When we are dealing with the structure we should initialize the structure member, because of structure member memory is allocated only when we creat a structure variable
- in Structure member yyou should not mention the storage type
struct Person person1;
person1.age = 25;
person1.height = 5.9;
Using Structures
Structures are incredibly useful for representing real-world entities in your programs. For instance, you could use a structure to represent a point in three-dimensional space, a record in a database, or even a complex data entity like a car.
Example: Using a Structure
Let's take an example of a structure to represent a point in a Cartesian coordinate system:
struct Point {
int x;
int y;
};
Now, you can create instances of this structure and use them to represent different points on a plane.
struct Point p1 = {2, 3};
struct Point p2 = {5, 7};
Simple program of Structures in C
#include<stdio.h>
struct one
{
int i;//structure member
char ch;//structure member
float f;//structure member
};// this semi column is mandtory
void main()
{
struct one s1;
s1.i=10;
s1.ch='a';
s1.f=23.5;
printf("%d %c %f\n",s1.i,s1.ch,s1.f);
}
Output: 10 a 23.500000
Conclusion
Structures in C provide a powerful tool for organizing and managing complex data in your programs. By grouping together related variables, you can create more organized and efficient code.
Top Resources
what is structure in C?
What is self-referential structure pointer in c?
What is structure padding? How to avoid structure padding?
differences between a structure and a union in c?
How can typedef be to define a type of structure in c?
Program to illustrate a function that assigns value to the structure
What is structure? What are differences between Arrays and Structures?
Write a program to find the sizeof structure without using sizeof operator?
Write a program that returns 3 numbers from a function using a structure in c.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!