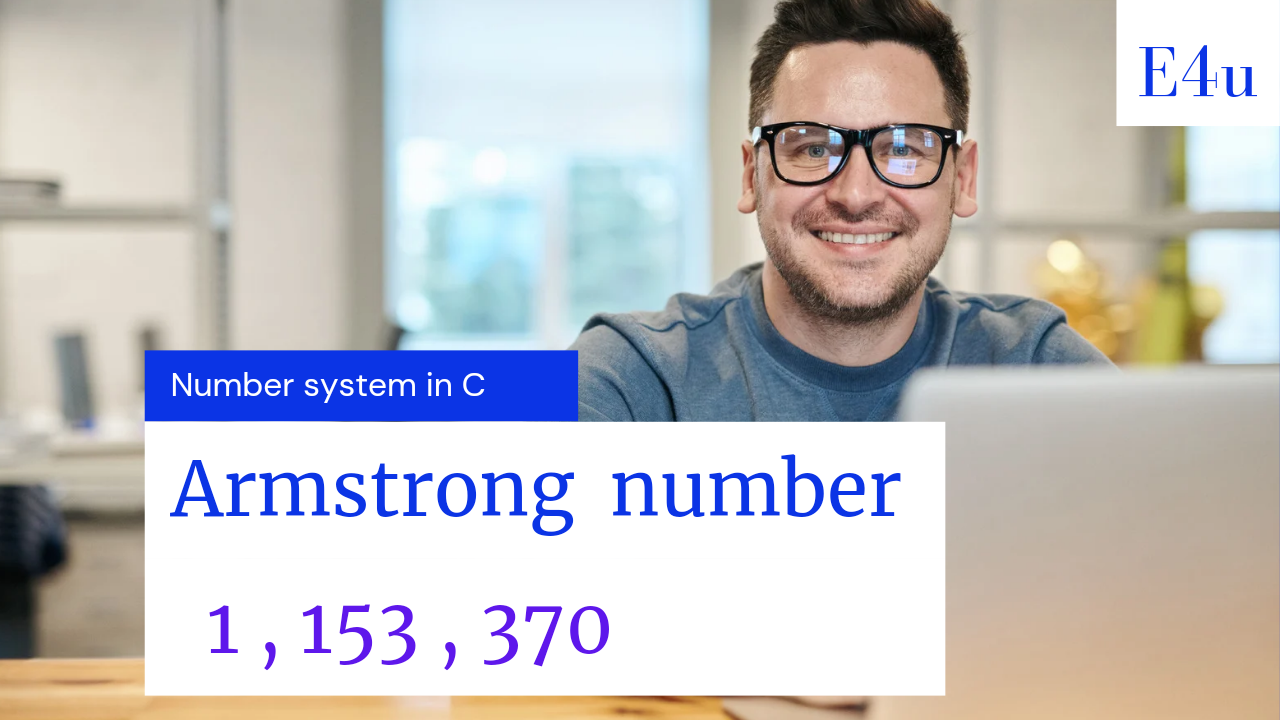
Armstrong Number in C programming
Armstrong Number in C Programming
Definition: An Armstrong number (also known as a narcissistic number, plenary number, or a plus perfect number) is a number that is equal to the sum of its own digits each raised to the power of the number of digits.
Example
For instance, 153 is an Armstrong number because 1^3 + 5^3 + 3^3 = 153.
How to Check for Armstrong Numbers in C Programming
Step 1: Get User Input
Use scanf or other input methods to get an integer from the user.
Step 2: Calculate the Number of Digits
Use a loop to count the number of digits in the input number.
Step 3: Calculate the Sum of Digits Raised to the Power of the Number of Digits
Use a loop to iterate through each digit and calculate the sum.
Step 4: Compare with the Original Number
Check if the sum calculated matches the original input.
Step 5: Display the Result
Print a message indicating whether the number is an Armstrong number or not.
Wrte a C Program To print Armstrong Number?
#include<stdio.h>
#include<stdlib.h>
int main() {
int number, originalNumber, remainder, result = 0, n = 0;
printf("Enter an integer: ");
scanf("%d", &number);
originalNumber = number;
// count the number of digits in the input number
while (originalNumber != 0) {
originalNumber /= 10;
++n;
}
originalNumber = number;
// calculate the sum of each digit raised to the power of the number of digits
while (originalNumber != 0) {
remainder = originalNumber % 10;
result += pow(remainder, n);
originalNumber /= 10;
}
// check whether the calculated result is equal to the input number
if (result == number)
printf("%d is an Armstrong number.", number);
else
printf("%d is not an Armstrong number.", number);
return 0;
}
Conclusion
In this program, we first take the input number from the user using scanf(). Then we count the number of digits in the input number using a while loop. Next, we calculate the sum of each digit raised to the power of the number of digits using another while loop and the pow() function from the math.h library. Finally, we check whether the calculated result is equal to the input number using an if statement and print the appropriate message to the user.
Armstrong numbers are interesting mathematical phenomena that can be easily checked using C programming.