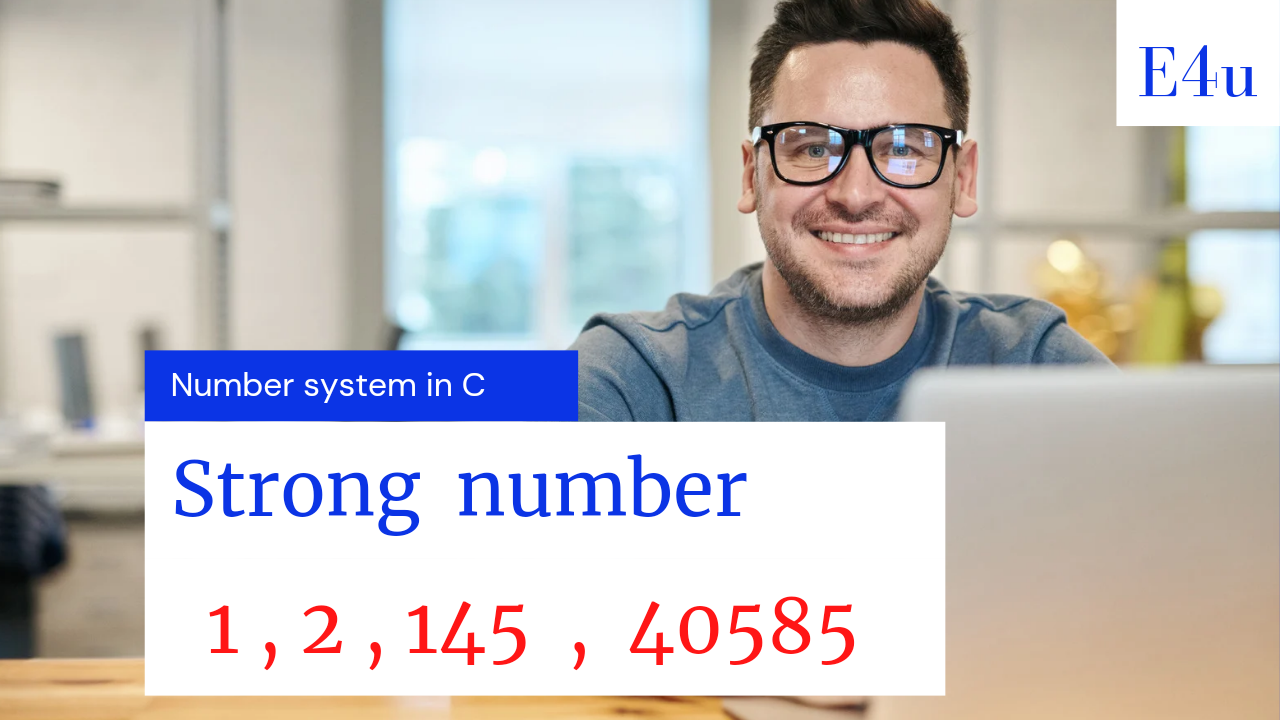
Strong number in c
Understanding Strong Numbers in C
What is a Strong Number?
- A strong number (also known as a factorial number) is a number that equals the sum of the factorial of its digits.
- For example, 145 is a strong number because 1! + 4! + 5! = 145.
How to Determine if a Number is Strong in C?
-
Calculate Factorials:
- Break down the number into its individual digits.
- Calculate the factorial of each digit.
-
Sum Factorials:
- Add up all the factorials calculated in the previous step.
-
Check Equality:
- Compare the sum obtained in step 2 with the original number.
- If they are equal, the number is strong.
Write a C Program for Strong Number?
#include
int factorial(int num) {
if (num == 0 || num == 1)
return 1;
else
return num * factorial(num - 1);
}
int isStrong(int num) {
int original = num;
int sum = 0;
while (num > 0) {
int digit = num % 10;
sum += factorial(digit);
num /= 10;
}
return (sum == original);
}
int main() {
int num;
printf("Enter a number: ");
scanf("%d", &num);
if (isStrong(num))
printf("%d is a strong number.", num);
else
printf("%d is not a strong number.", num);
return 0;
}
Usage:
- Copy the provided C code into your preferred C compiler.
- Input a number to check if it's a strong number.
Conclusion:
- Strong numbers are an interesting mathematical concept in programming.
- This C program efficiently determines whether a given number is strong or not.