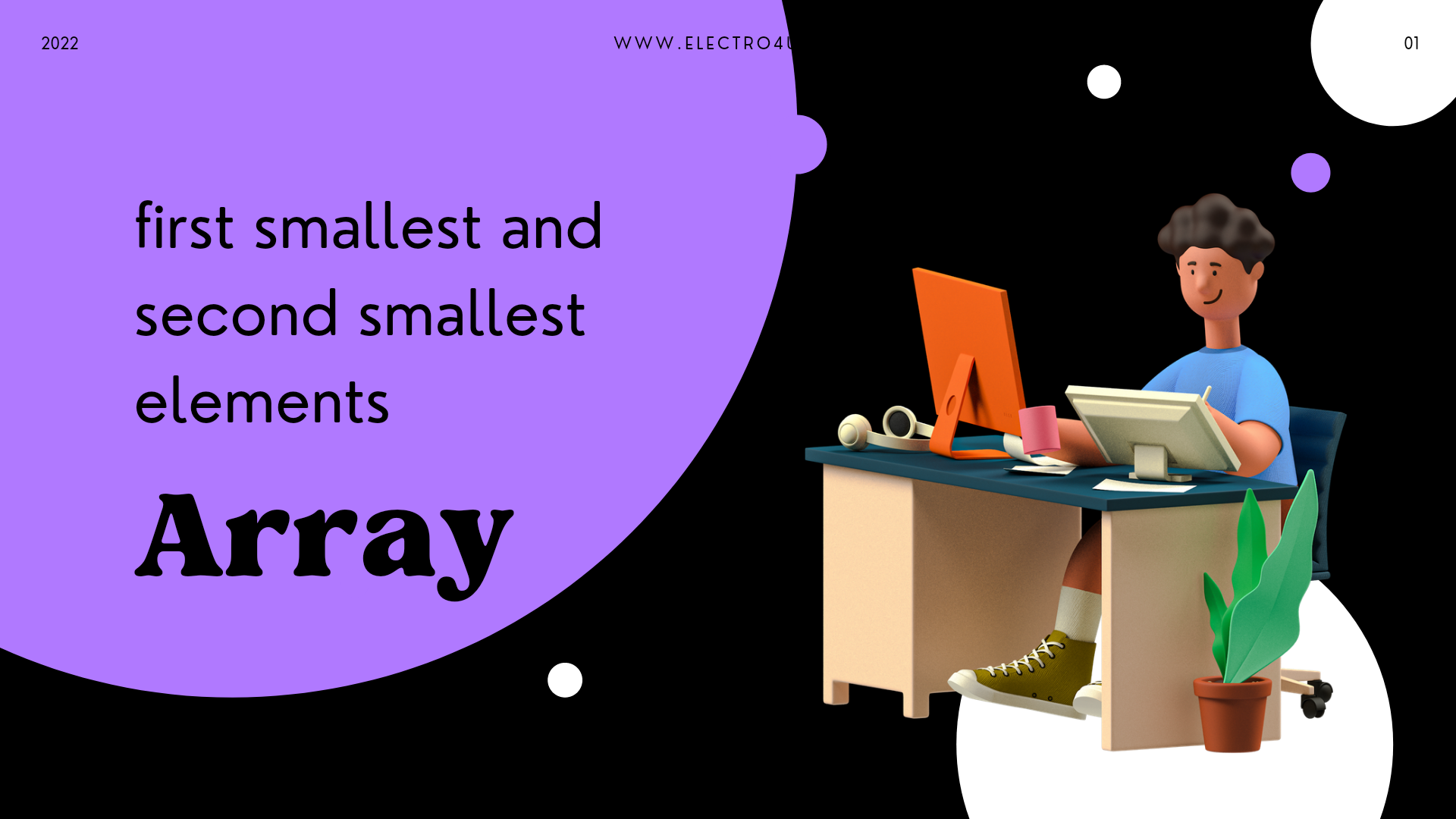
write a program to find the first smallest and second smallest elements of a given array.
Program in C to Find the First and Second Smallest Elements of an Array
"Write a C program to find the first smallest and second smallest elements of a given array"
means that you need to create a computer program using the C programming language that takes an array as input and outputs the first smallest and second smallest elements in the array.
The program should first ask the user to input the size of the array and then the elements of the array. Then the program should iterate through the array to find the first smallest element, and then iterate through the array again to find the second smallest element, excluding the first smallest element. Finally, the program should output the first smallest and second smallest elements to the console.
Overall, the goal of the program is to provide a solution to the problem of finding the two smallest elements in an array using the C programming language.
Program in C to find the first and second smallest elements in an array
#include
int main() {
int arr[100], n, i, first, second;
printf("Enter the size of the array: ");
scanf("%d", &n);
printf("Enter the elements of the array: ");
for (i = 0; i < n; i++) {
scanf("%d", &arr[i]);
}
// Find the first smallest element
first = arr[0];
for (i = 1; i < n; i++) {
if (arr[i] < first) {
first = arr[i];
}
}
// Find the second smallest element
second = arr[0];
for (i = 1; i < n; i++) {
if (arr[i] < second && arr[i] != first) {
second = arr[i];
}
}
printf("The first smallest element is %d\n", first);
printf("The second smallest element is %d\n", second);
return 0;
}
Solution: In this program, the user is asked to input the size and elements of the array. The program then finds the first smallest element by iterating through the array and comparing each element to the first element. The second smallest element is found by iterating through the array again and comparing each element to the second element, but only if it is not equal to the first smallest element. The first and second smallest elements are then printed to the console.
Further Reading:
write a program to find the largest elements of a given array with an index.
write a c program to find the second largest elements of a given array.
write a program to find the first largest and second-largest elements of a given array.
Write a c program find largest elements in this array number or index
Write a C program to find out the second largest and second smallest elements of any unsorted array without using any shorting Technique
write a program to find the smallest elements of a given array with an index.
write a program to find the first largest and first smallest elements of a given array.
write a program to find the second smallest elements of a given array.
write a program to find the second largest and second smallest elements of a given array.
write a program to find the first smallest and second smallest elements of a given array.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!