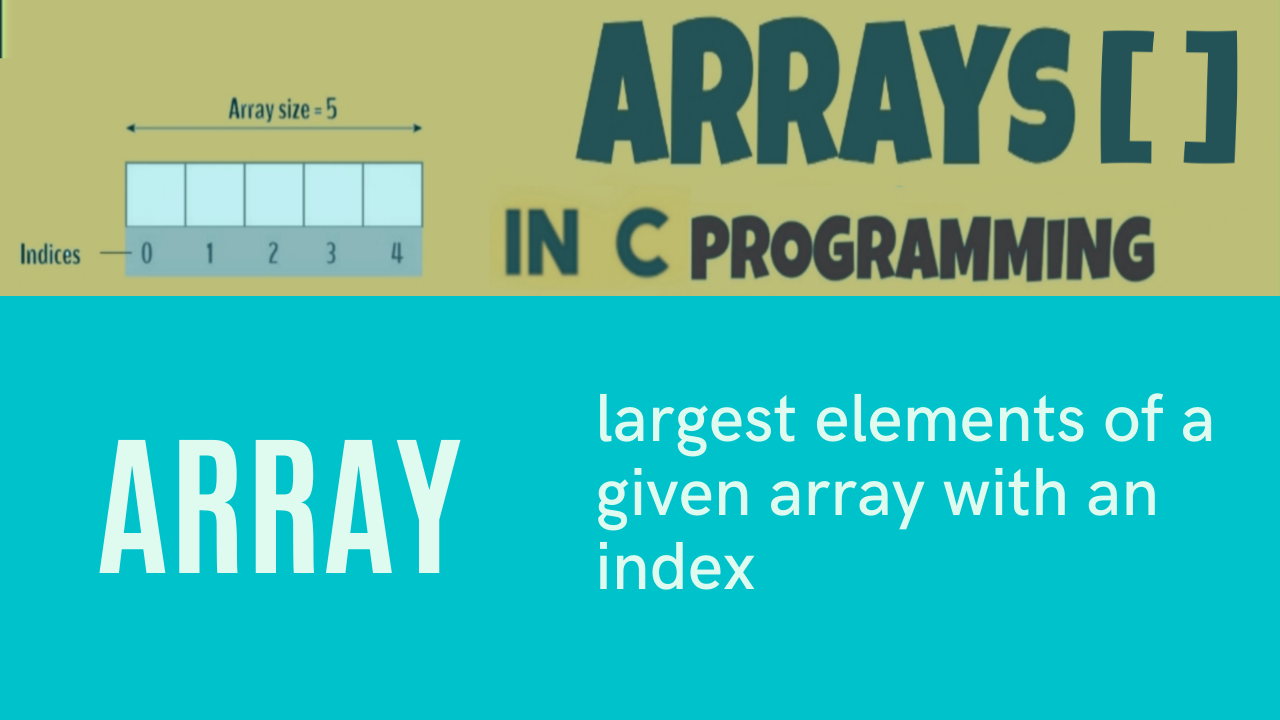
write a program to find the largest elements of a given array with an index.
Write C a program to find the largest elements of a given array with an index.
Writing a C program to find the largest element of a given array with an index means creating a program in the C programming language that takes an array of integers as input, finds the largest element in the array, and returns the index of that largest element.
To do this, we can initialize two variables: max_elem
and max_index.
We can then loop through the array, comparing each element to max_elem
. If the current element is greater than max_elem
, we update max_elem
to the current element and max_index
to the current index.
At the end of the loop, max_elem
should contain the largest element in the array, and max_index
should contain its index. We can then output these values to the user.
Find the Largest Element of an Array with Its Index in C
#include<stdio.h>
int main() {
int arr[] = {10, 20, 30, 40, 50};
int n = sizeof(arr) / sizeof(arr[0]);
int max_elem = arr[0];
int max_index = 0;
// Find the largest element and its index
for (int i = 0; i < n; i++) {
if (arr[i] > max_elem) {
max_elem = arr[i];
max_index = i;
}
}
// Print the largest element and its index
printf("The largest element is %d and its index is %d\n", max_elem, max_index);
return 0;
}
Output of the C program you provided is:
The largest element is 50 and its index is 4
This is because the largest element in the array arr is 50
, and it is located at index 4.
In this program, we first declare an integer array arr
and initialize it with some values. We then calculate the size of the array and initialize max_elem
to the first element of the array and max_index
to 0.
We then loop through the array using a for
loop and compare each element to max_elem.
If the current element is larger than max_elem,
we update max_elem
and max_index
accordingly. Finally, we print
the largest element and its index using the printf function.
This program demonstrates how to find the largest element of an array along with its index using C.
Further Reading:
write a program to find the largest elements of a given array with an index.
write a c program to find the second largest elements of a given array.
write a program to find the first largest and second-largest elements of a given array.
Write a c program find largest elements in this array number or index
Write a C program to find out the second largest and second smallest elements of any unsorted array without using any shorting Technique
write a program to find the smallest elements of a given array with an index.
write a program to find the first largest and first smallest elements of a given array.
write a program to find the second smallest elements of a given array.
write a program to find the second largest and second smallest elements of a given array.
write a program to find the first smallest and second smallest elements of a given array.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!