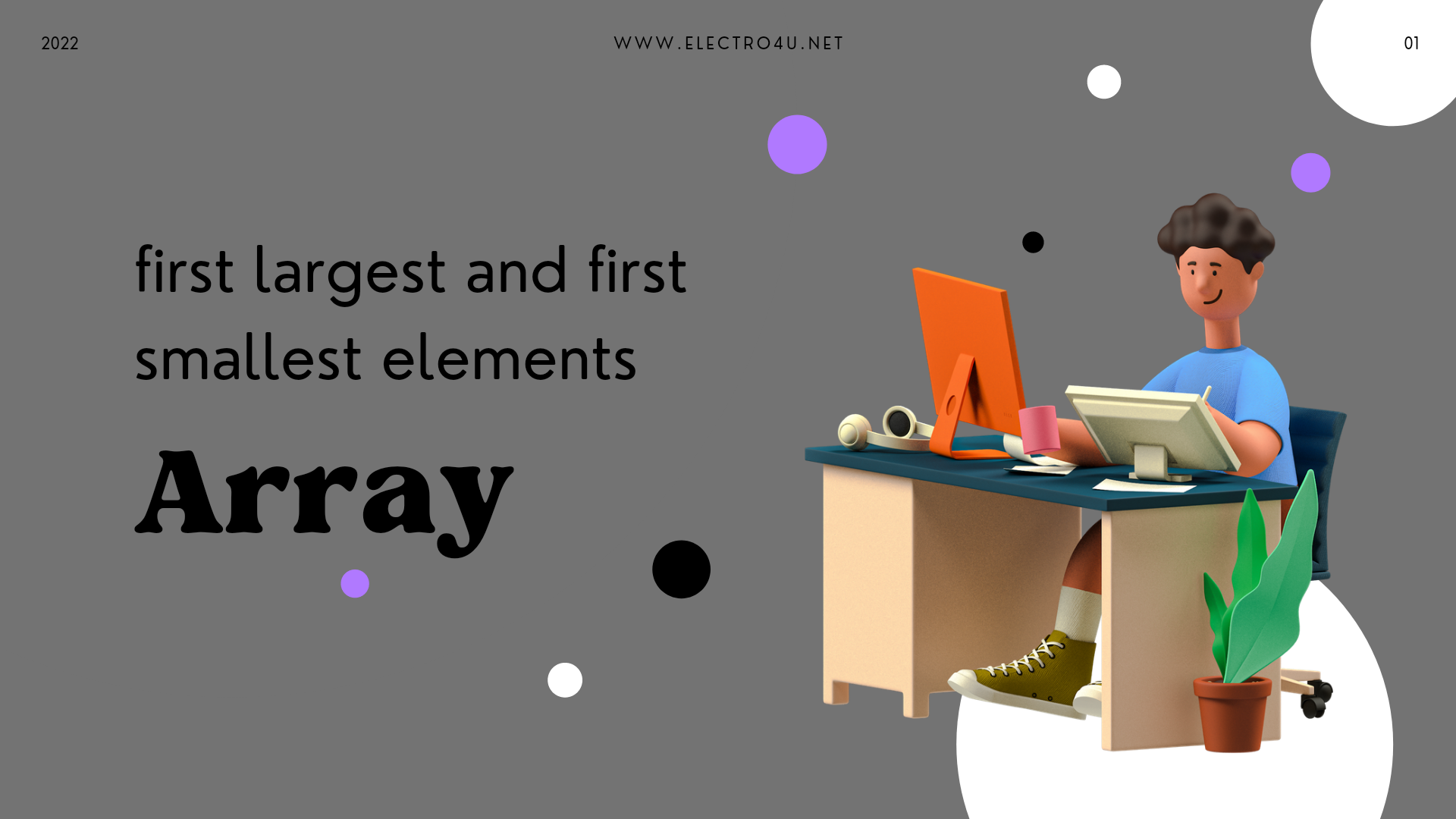
write a C program to find the first largest and first smallest elements of a given array.
C Program to Find First Largest and First Smallest Elements in an Array
When you are asked to write a C program to find the first largest and first smallest elements of a given array, it means that you are required to create a program that takes an array of integers as input and finds the first largest and first smallest elements of that array.
"First largest" refers to the largest element in the array that appears first in the sequence. Similarly, "first smallest" refers to the smallest element in the array that appears first in the sequence.
For example, if we have an array arr
with the following elements: {10, 5, 20, 3, 30, 40, 1},
the first largest element is 20 (because it appears first among the largest elements in the array), and the first smallest element is 5 (because it appears first among the smallest elements in the array).
So, your C program should be able to determine the first largest and first smallest elements of a given array, and print them out as output.
C program that finds the first largest and first smallest elements of a given array:
#include<stdio.h>
int main() {
int arr[] = {10, 20, 30, 40, 50}; // sample array
int size = sizeof(arr) / sizeof(int); // get size of the array
int i, first_largest, first_smallest;
// initialize first_largest and first_smallest to the first element of the array
first_largest = first_smallest = arr[0];
// loop through the array
for (i = 1; i < size; i++) {
// check if the current element is larger than the current first_largest
if (arr[i] > first_largest) {
first_largest = arr[i];
break;
}
// check if the current element is smaller than the current first_smallest
if (arr[i] < first_smallest) {
first_smallest = arr[i];
break;
}
}
printf("The first largest element is: %d\n", first_largest);
printf("The first smallest element is: %d\n", first_smallest);
return 0;
}
In this program, we first declare an array arr
with some sample values, and then calculate its size using sizeof(arr) / sizeof(int).
We then use a for loop to iterate over the elements of the array, starting from the second element (since the first element
is already assigned to first_largest
and first_smallest).
Within the loop, we check if the current element is larger than the current first_largest
element. If it is, we update first_largest
to the current element. Similarly, we check if the current element is smaller than the current first_smallest
element. If it is, we update first_smallest
to the current element.
Finally, we print out the values of first_largest
and first_smallest.
Note that we use a break
statement after updating first_largest
and first_smallest
to ensure that we only find the first occurrence of each in the array.
Further Reading:
write a program to find the largest elements of a given array with an index.
write a c program to find the second largest elements of a given array.
write a program to find the first largest and second-largest elements of a given array.
Write a c program find largest elements in this array number or index
Write a C program to find out the second largest and second smallest elements of any unsorted array without using any shorting Technique
write a program to find the smallest elements of a given array with an index.
write a program to find the first largest and first smallest elements of a given array.
write a program to find the second smallest elements of a given array.
write a program to find the second largest and second smallest elements of a given array.
write a program to find the first smallest and second smallest elements of a given array.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!