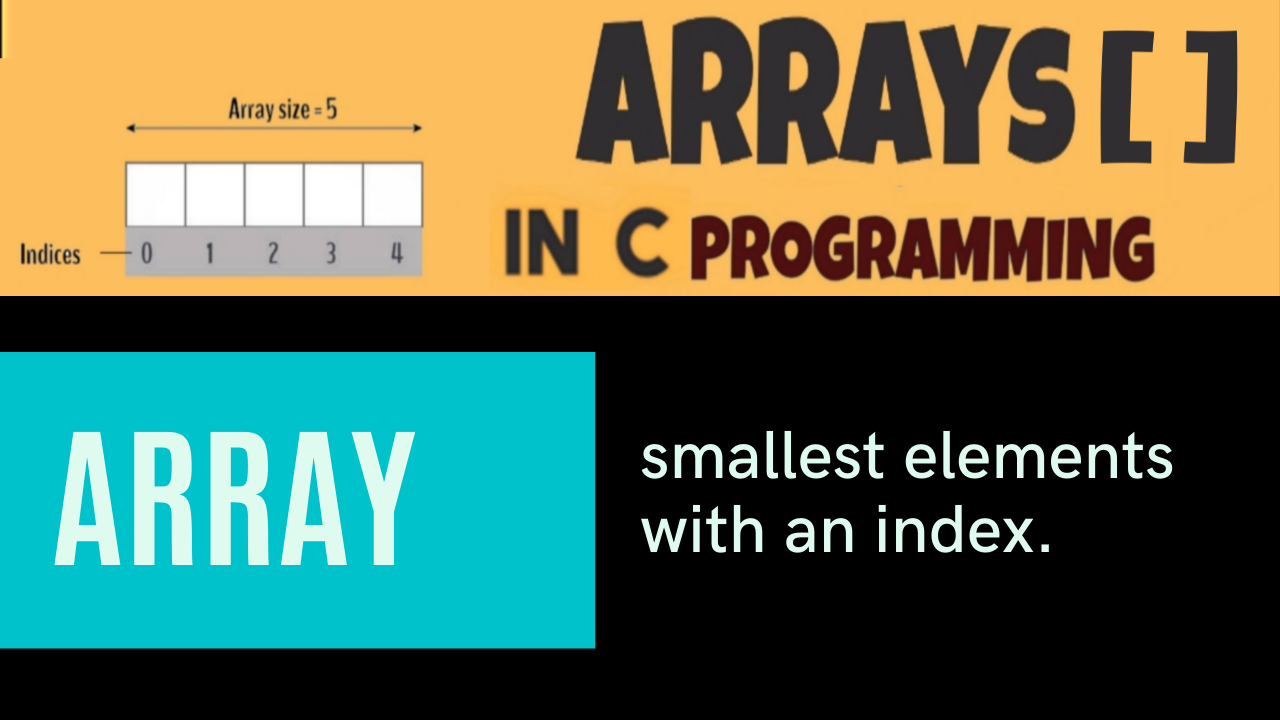
write a program to find the smallest elements of a given array with an index in c
Find the Smallest Element of an Array with Its Index in C
Writing a C program to find the smallest element of a given array with its index means creating a program in the C programming language that can read an array of integers as input and then determine the smallest value in the array along with its index. The program should be able to iterate through the array elements, compare them with each other, and keep track of the index of the smallest element. Finally, the program should print out the smallest element of the array and its index to the console.
C to find the smallest element of a given array along with its index:
C programming
#include
int main() {
int arr[] = {10, 20, 30, 40, 5};
int min_elem = arr[0]; // initialize min_elem to first element of array
int min_index = 0; // initialize min_index to 0
int size = sizeof(arr) / sizeof(arr[0]);
// Find the smallest element and its index
for (int i = 0; i < size; i++) {
if (arr[i] < min_elem) {
min_elem = arr[i];
min_index = i;
}
}
// Print the smallest element and its index
printf("The smallest element is %d and its index is %d\n", min_elem, min_index);
return 0;
}
Output of the C program you provided is:
The smallest element is 5 and its index is 4
This is because the smallest element in the array arr is 5,
and it is located at index 4.
In this program, we first initialize min_elem
to the first element of the array and min_index
to 0.
We then loop through the array using a for
loop and compare each element to min_elem
. If the current element is smaller than min_elem,
we update min_elem
and min_index
accordingly. Finally, we print the smallest element and its index.
This program demonstrates how to find the smallest element of an array along with its index using C.
Further Reading:
write a program to find the largest elements of a given array with an index.
write a c program to find the second largest elements of a given array.
write a program to find the first largest and second-largest elements of a given array.
Write a c program find largest elements in this array number or index
Write a C program to find out the second largest and second smallest elements of any unsorted array without using any shorting Technique
write a program to find the smallest elements of a given array with an index.
write a program to find the first largest and first smallest elements of a given array.
write a program to find the second smallest elements of a given array.
write a program to find the second largest and second smallest elements of a given array.
write a program to find the first smallest and second smallest elements of a given array.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!