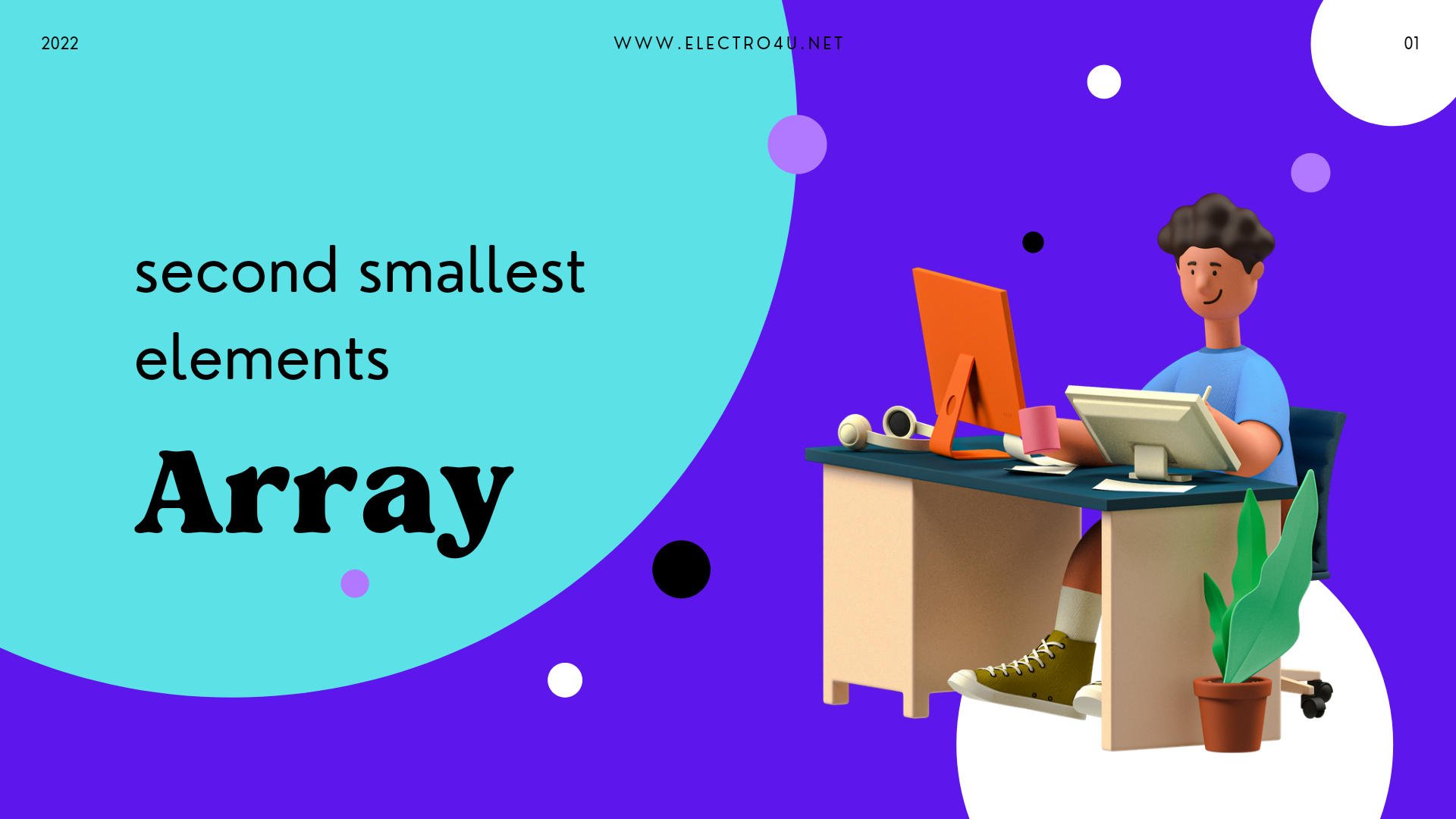
write a C Program to find the second smallest elements of a given array.
How to Find the Second Smallest Element in an Array: A Step-by-Step Guide
Write a C program to find the second smallest element of a given array" means that you need to write a computer program using the C programming language that takes an array of integers as input and outputs the second smallest element of that array.
For example, if the given array is [4, 7, 2, 9, 5],
the second smallest element is 4, so the program should output 4.
To solve this problem, the program needs to compare each element of the array with the other elements and find the smallest and second smallest elements. Once it has found the second smallest element, it should output that element to the user.
C program that finds the second smallest element in a given array:
#include
int main() {
int arr[] = {4, 2, 6, 1, 8, 5}; // example array
int n = sizeof(arr) / sizeof(arr[0]); // size of array
int smallest = arr[0]; // initialize smallest element to first element
int second_smallest = arr[0]; // initialize second smallest element to first element
for (int i = 0; i < n; i++) {
if (arr[i] < smallest) {
second_smallest = smallest; // update second smallest element
smallest = arr[i]; // update smallest element
}
else if (arr[i] < second_smallest && arr[i] != smallest) {
second_smallest = arr[i]; // update second smallest element
}
}
printf("The second smallest element in the array is: %d", second_smallest);
return 0;
}
Solution: This program uses a loop to iterate through the array and update the values of the smallest and second smallest elements as it goes. It then prints out the value of the second smallest element at the end. Note that if the array has fewer than two elements, this program will not work correctly.
Further Reading:
write a program to find the largest elements of a given array with an index.
write a c program to find the second largest elements of a given array.
write a program to find the first largest and second-largest elements of a given array.
Write a c program find largest elements in this array number or index
Write a C program to find out the second largest and second smallest elements of any unsorted array without using any shorting Technique
write a program to find the smallest elements of a given array with an index.
write a program to find the first largest and first smallest elements of a given array.
write a program to find the second smallest elements of a given array.
write a program to find the second largest and second smallest elements of a given array.
write a program to find the first smallest and second smallest elements of a given array.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!