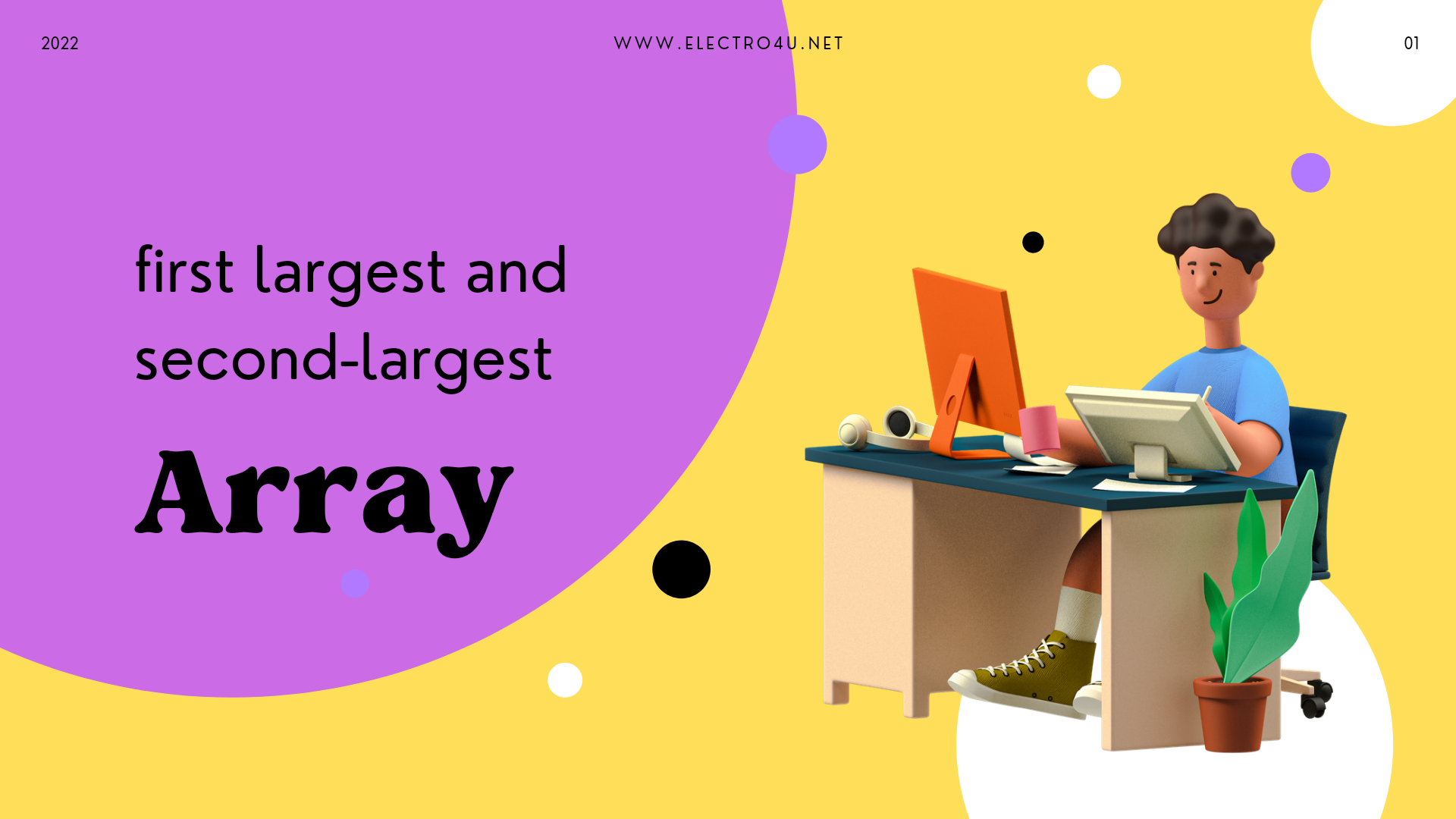
write a program to find the first largest and second-largest elements of a given array.
Find the Largest and Second-Largest Elements in an Array with a C Program
"Write a C program to find the first largest and second-largest elements of a given array"
means that you need to write a program in the C programming language that takes an array of integers as input and finds the largest and second-largest elements in the array. The program should output the values of the largest and second-largest elements.
To solve this problem, you need to iterate through the array and keep track of the largest and second-largest elements you've seen so far. You can do this by initializing two variables to very small values, and then updating them as you iterate through the array. Once you've finished iterating through the array, you can output the values of the two variables you've been keeping track of.
C- Program to find the first and second-largest elements in an array:
C programming
#include
void find_largest(int arr[], int n) {
int max1 = INT_MIN, max2 = INT_MIN;
for (int i = 0; i < n; i++) {
if (arr[i] > max1) {
max2 = max1;
max1 = arr[i];
}
else if (arr[i] > max2 && arr[i] != max1) {
max2 = arr[i];
}
}
printf("The first largest element is %d\n", max1);
printf("The second largest element is %d\n", max2);
}
int main() {
int arr[] = {3, 5, 1, 8, 4, 2};
int n = sizeof(arr)/sizeof(arr[0]);
find_largest(arr, n);
return 0;
}
This program uses two variables max1
and max2
to keep track of the largest and second-largest
elements in the array. It iterates through the array, updating max1 and max2 as necessary, and then prints the results.
output
The first largest element is 8
The second largest element is 5
Further Reading:
write a program to find the largest elements of a given array with an index.
write a c program to find the second largest elements of a given array.
write a program to find the first largest and second-largest elements of a given array.
Write a c program find largest elements in this array number or index
Write a C program to find out the second largest and second smallest elements of any unsorted array without using any shorting Technique
write a program to find the smallest elements of a given array with an index.
write a program to find the first largest and first smallest elements of a given array.
write a program to find the second smallest elements of a given array.
write a program to find the second largest and second smallest elements of a given array.
write a program to find the first smallest and second smallest elements of a given array.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!