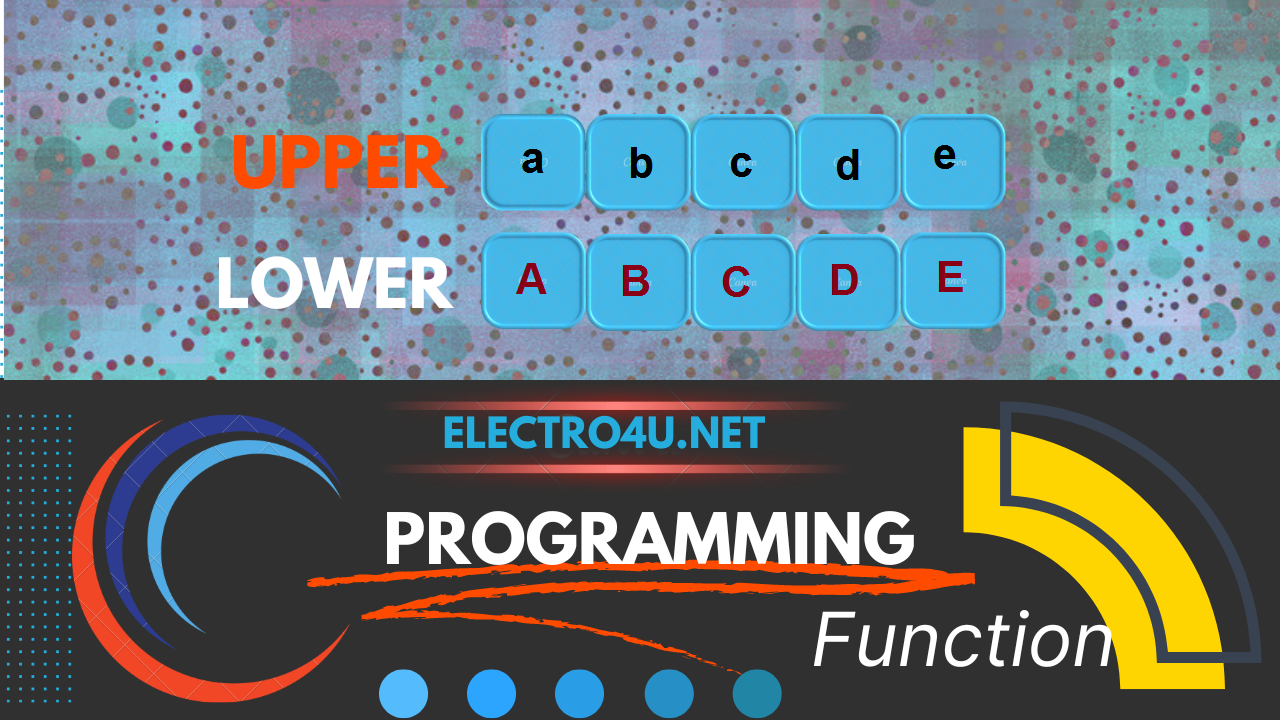
C Function to Convert a String to Uppercase: Design Guide
Uppercase String Conversion in C: Step-by-Step Function Design
"Design a function to convert given string into upper character using own function"
means to create a function in the C programming language that can take a string as input and convert all of its characters to uppercase using your own implementation, without relying on any built-in functions or libraries.
To design a function to convert a given string to upper case in C, we can use the following approach:
Define a function with the following signature:
char *touppercase(char *string);
The function will take a pointer to the string to be converted as input and return a pointer to the converted string as output.
- Inside the function, iterate over the string character by character.
- For each character, check if it is a lowercase letter. If it is, convert it to the corresponding uppercase letter by subtracting 32 from its ASCII value.
- Return the pointer to the converted string.
Complete implementation of the touppercase() function:
#include <stdio.h>
#include <string.h>
char *touppercase(char *string) {
char *ptr = string;
while (*ptr != '\0') {
if (*ptr >= 'a' && *ptr <= 'z') {
*ptr -= 32;
}
ptr++;
}
return string;
}
To use the touppercase()
function, simply pass a pointer to the string you want to convert to the function as input. The function will return a pointer to the converted string.
Example of how to use the touppercase() function:
char string[] = "This is a test string.";
// Convert the string to upper case.
char *uppercase_string = touppercase(string);
// Print the converted string.
printf("%s\n", uppercase_string);
Output:
THIS IS A TEST STRING.
Further Reading:
Implementation of strncat() in C
add source string at the end of destination string
comparing two string using strcmp and strncmp
Design a function to copy to copy string into destination string using strcpy and strncpy
how to implement implementation of strncpy in c
Design a function to calculate the length of the string using strlen
pre defined string based function
Design a function using my_strcpy and using my_strchr we need to select given char in given string
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!