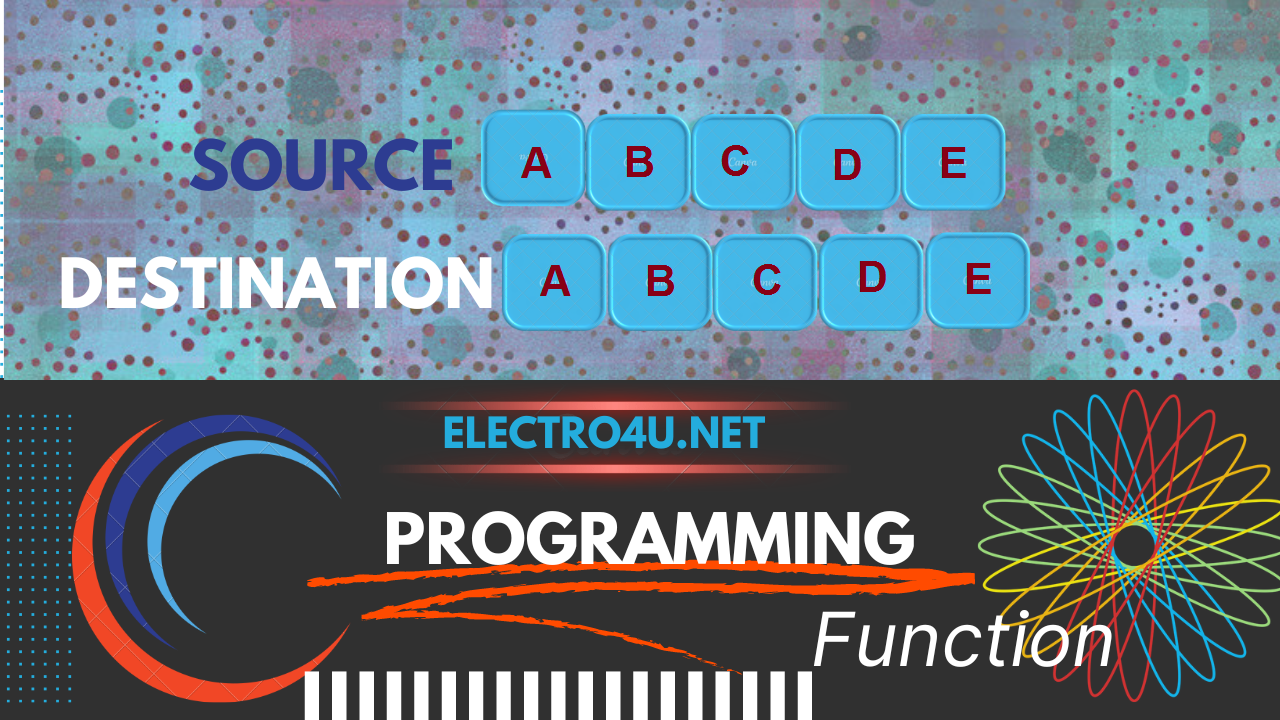
Design a function to source string into destination string
Copying Strings in C: A Step-by-Step Guide
To design a function to source string into destination string in C, we can use the following steps:
- Declare the function prototype. The function prototype should specify the name of the function, the return type, and the parameter types.
- Implement the function body. The function body should copy the characters from the source string to the destination string.
- Return the destination string from the function.
Sample function to source string into destination string in C:
C Programming
#include <string.h>
char *source_string_into_destination_string(char *destination_string, const char *source_string) {
// Copy the characters from the source string to the destination string.
strcpy(destination_string, source_string);
// Return the destination string.
return destination_string;
}
To use the function, we would first declare a destination string. Then, we would call the function, passing in the destination string and the source string as arguments. The function would copy the characters from the source string to the destination string and return the destination string.
How to use the function:
C Programming
#include <stdio.h>
#include <string.h>
int main() {
// Declare a destination string.
char destination_string[100];
// Call the function to source string into destination string.
char *result = source_string_into_destination_string(destination_string, "This is a test string.");
// Print the destination string.
printf("The destination string is: %s\n", destination_string);
return 0;
}
Output:
The destination string is: This is a test string.
Further Reading:
Implementation of strncat() in C
add source string at the end of destination string
comparing two string using strcmp and strncmp
Design a function to copy to copy string into destination string using strcpy and strncpy
how to implement implementation of strncpy in c
Design a function to calculate the length of the string using strlen
pre defined string based function
Design a function using my_strcpy and using my_strchr we need to select given char in given string
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!