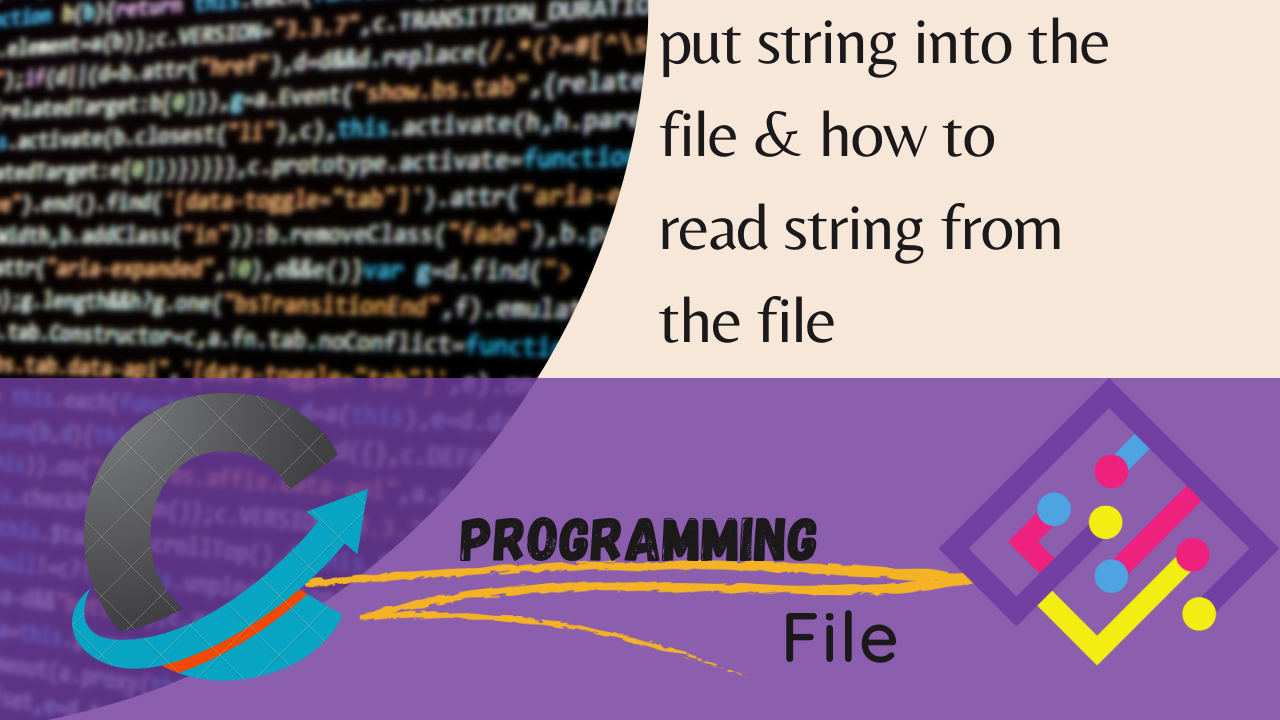
How to put string into the file and how to read string from the file in c
Working with Strings in Files in C
Putting a String into a File
-
Open the File: Use fopen() to open the file in the desired mode (e.g., write mode "w" for putting data into the file).
c-programmingFILE *filePointer; filePointer = fopen("example.txt", "w");
-
Write String to File: Use fprintf() to write a string to the file.
c-programmingfprintf(filePointer, "Hello, this is a sample string.");
-
Close the File: Always close the file after writing.
c-programmingfclose(filePointer);
Reading a String from a File
-
Open the File: Use fopen() to open the file in the desired mode (e.g., read mode "r").
c-programmingFILE *filePointer; filePointer = fopen("example.txt", "r");
-
Read String from File: Use fscanf() to read a string from the file.
c-programmingchar buffer[100]; // Assuming a maximum string length of 100 fscanf(filePointer, "%s", buffer);
-
Close the File: Always close the file after reading.
c-programming
fclose(filePointer);
Putting a string into a file:
c-programming
FILE *filePointer;
filePointer = fopen("example.txt", "w");
fprintf(filePointer, "Hello, this is a sample string.");
fclose(filePointer);
Reading a string from a file:
c-programming
FILE *filePointer;
filePointer = fopen("example.txt", "r");
char buffer[100];
fscanf(filePointer, "%s", buffer);
fclose(filePointer);
File Operations in C: Writing and Reading Strings
#include <stdio.h>
int main() {
FILE *filePointer;
char textToWrite[100]; // Assuming a string of up to 100 characters
char buffer[100]; // Assuming a buffer of up to 100 characters
// Putting a String into a File
filePointer = fopen("example.txt", "w");
if (filePointer == NULL) {
printf("Error opening file for writing.\n");
return -1; // Return an error code
}
printf("Enter a string to write to the file: ");
gets(textToWrite); // Note: gets() is used for simplicity, not recommended in real code
fprintf(filePointer, "%s", textToWrite);
fclose(filePointer);
// Reading a String from a File
filePointer = fopen("example.txt", "r");
if (filePointer == NULL) {
printf("Error opening file for reading.\n");
return -1; // Return an error code
}
fgets(buffer, sizeof(buffer), filePointer);
fclose(filePointer);
printf("String read from file: %s", buffer);
return 0;
}
Output
Enter a string to write to the file: Hello, World!
String read from file: Hello, World!
Conclusion:
- You've learned how to put a string into a file and how to read a string from a file in C.
- These file I/O operations are fundamental for many real-world applications.
Note:
- Error handling is crucial when working with files in C. Always check the return values of file-related functions for errors.
- Ensure that you have the necessary permissions to access and modify the file you're working with.
Further Reading:
For further information and examples, Please visit[ C-Programming From Scratch to Advanced 2023-2024]
Top Resources
- Learn-C.org
- C Programming at LearnCpp.com
- GeeksforGeeks - C Programming Language
- C Programming on Tutorialspoint
- Codecademy - Learn C
- CProgramming.com
- C Programming Wikibook
- C Programming - Reddit Community
- C Programming Language - Official Documentation
- GitHub - Awesome C
- HackerRank - C Language
- LeetCode - C