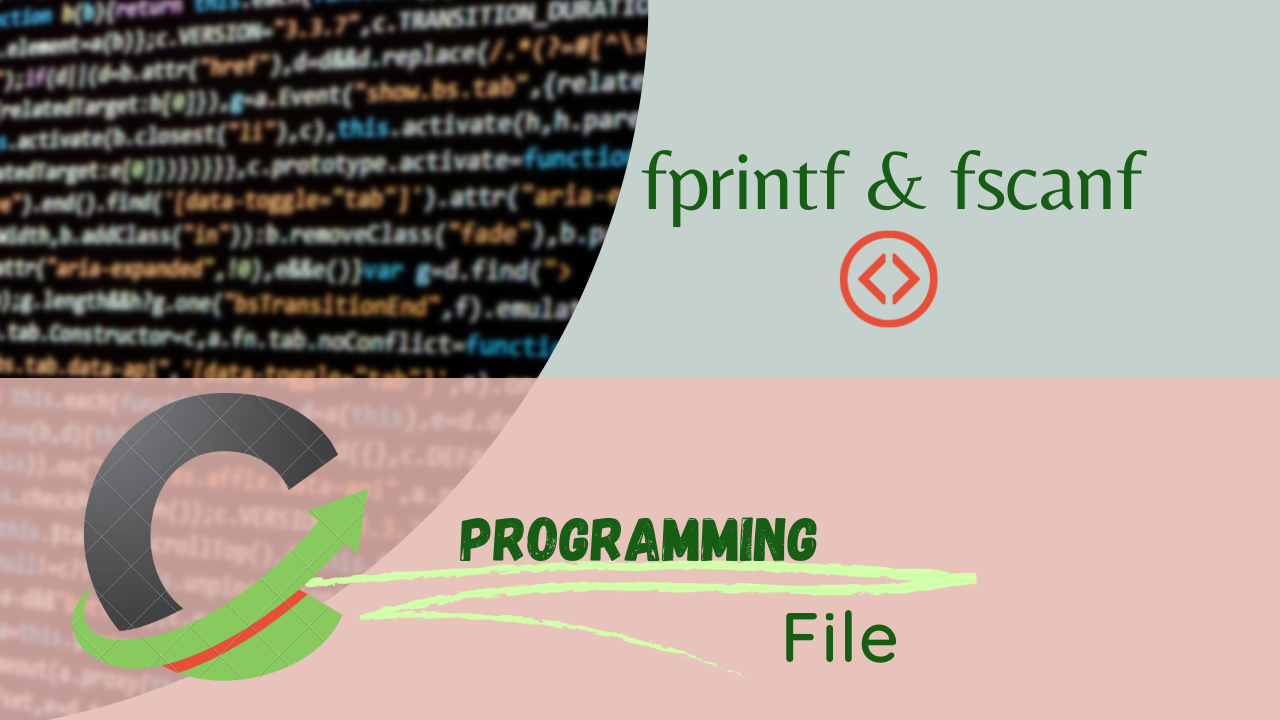
fprintf and fscanf in c with electro4u.net
What is fprintf?
fprintf and fscanf are two functions in the C programming language that are used for reading from and writing to files. or fprintf is used to write formatted data to a file
fprintf Function
fprintf stands for "file print formatted." It is used to write formatted output to a file.
syntax of fprintf is:
- stream: This is a pointer to the file where the output is to be written.
- format: This is a string that contains format specifiers similar to printf.
- ...: These are the values to be formatted and written to the file.
Example Usage of fprintf:
#include <stdio.h>
int main() {
FILE *file;
file = fopen("output.txt", "w");
if (file == NULL) {
printf("Error opening file.\n");
return 1;
}
fprintf(file, "Hello, World!\n");
fprintf(file, "The value of pi is approximately %.2f\n", 3.14);
fclose(file);
return 0;
}
In this example, a file named output.txt is opened in write mode. Two lines of text are written to the file using fprintf, and then the file is closed.
Print the Data into the file using fprintf in c
#include
void main()
{
int i=123;
FILE *fp;
fp=fopen("data","w");
fprintf(fp,"%d",i);
}
Reverse Program- Print the Data into the file using fprintf in c
#include<stdio.h>
void main()
{
int i;
FILE *fp=fopen("data","r");
fscanf(fp,"%d",&i);
fprintf("i=%d\n",i);
}
fscanf Function:
fscanf stands for "file scan formatted." It is used to read formatted input from a file.
syntax of fscanf is:
int fscanf(FILE *stream, const char *format, ...);
- stream: This is a pointer to the file from which the input is to be read.
- format: This is a string that contains format specifiers similar to scanf.
- ...: These are pointers to the variables where the values will be stored.
Example Usage of fscanf:
#include <stdio.h>
int main() {
FILE *file;
char str[50];
float pi;
file = fopen("input.txt", "r");
if (file == NULL) {
printf("Error opening file.\n");
return 1;
}
fscanf(file, "%s", str);
fscanf(file, "%f", &pi);
printf("String: %s\n", str);
printf("Value of pi: %.2f\n", pi);
fclose(file);
return 0;
}
In this example, a file named input.txt is opened in read mode. The first line of the file is read into the str variable, and the second line (which should be a floating-point number) is read into the pi variable using fscanf.
Remember to always check if the file was opened successfully before attempting to read from or write to it.
Summary:
- fprintf is used for writing to a file, providing formatted output.
- fscanf is used for reading from a file, providing formatted input.
Both functions work with pointers to FILE structures, which represent the file being read from or written to. Always remember to open and close files properly and handle potential errors.
Further Reading:
For further information and examples, Please visit[ C-Programming From Scratch to Advanced 2023-2024]
Top Resources
- Learn-C.org
- C Programming at LearnCpp.com
- GeeksforGeeks - C Programming Language
- C Programming on Tutorialspoint
- Codecademy - Learn C
- CProgramming.com
- C Programming Wikibook
- C Programming - Reddit Community
- C Programming Language - Official Documentation
- GitHub - Awesome C
- HackerRank - C Language
- LeetCode - C