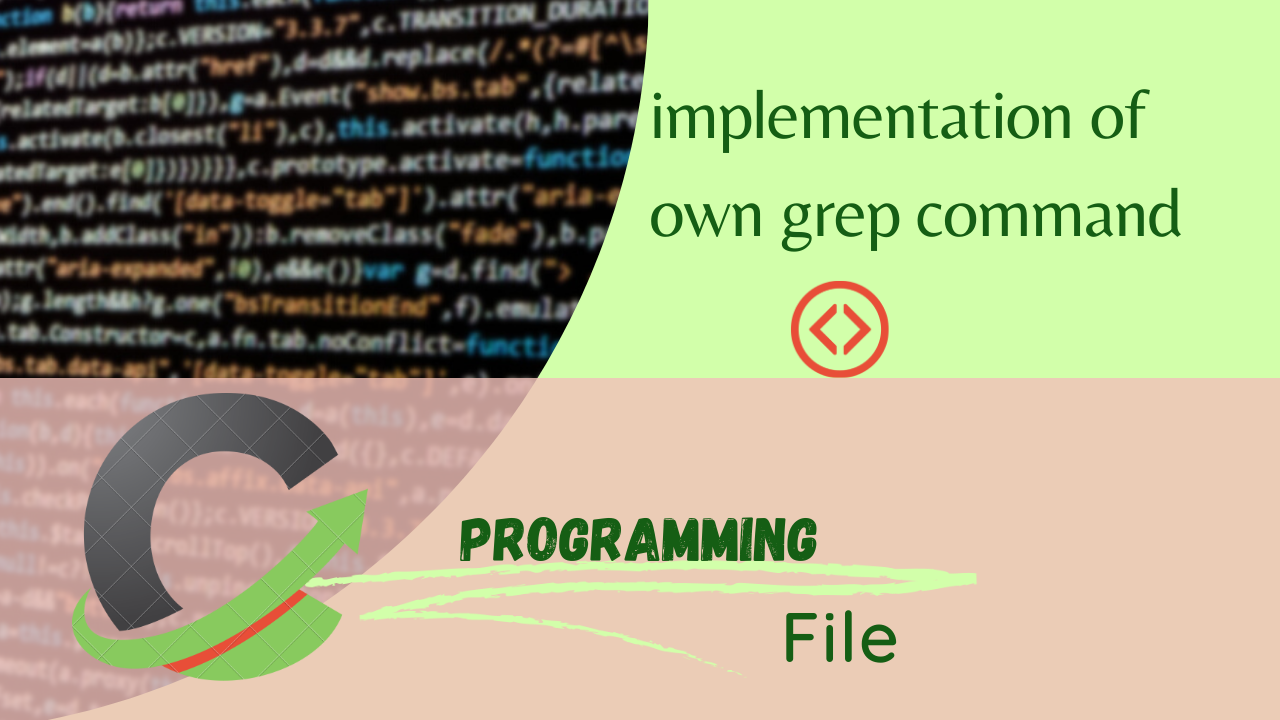
implementation of own grep command in c with electro4u
What is the grep command in the c programming language
The grep command is a powerful tool used to search for a specific string or pattern in a file or multiple files.
Syntax of grep command
implementation of the grep command in C using file:
There is no built-in grep command in the C programming language. However, it is possible to implement a simple version of grep in C using the following steps:
- Open the file to be searched using the fopen() function.
- Read each line of the file using the fgets() function.
- Search for the pattern in the line using the strstr() function.
- If the pattern is found, print the line to the console.
- Close the file using the fclose() function.
Example 01: C program to implement a simple version of grep:
#include <stdio.h>
#include <string.h>
int main(int argc, char *argv[]) {
// Check the number of arguments.
if (argc != 3) {
printf("Usage: %s <pattern> <filename>\n", argv[0]);
return 1;
}
// Open the file to be searched.
FILE *fp = fopen(argv[2], "r");
if (fp == NULL) {
printf("Error opening file: %s\n", argv[2]);
return 1;
}
// Read each line of the file and search for the pattern.
char line[1024];
while (fgets(line, sizeof(line), fp) != NULL) {
if (strstr(line, argv[1]) != NULL) {
printf("%s", line);
}
}
// Close the file.
fclose(fp);
return 0;
}
This program can be compiled using the following command:
gcc -o grep grep.c
To use the program, simply pass the pattern to search for and the filename to search in as arguments. For example, to search for the pattern "hello" in the file "myfile.txt", you would use the following command:
./grep hello myfile.txt
This would print all lines in the file that contain the pattern "hello"
Example 02: C program to implement of own grep command using file and micros
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#define MAX_LINE_LENGTH 1024
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: %s \n", argv[0]);
exit(1);
}
char *pattern = argv[1];
char *filename = argv[2];
FILE *fp;
char line[MAX_LINE_LENGTH];
int line_number = 1;
int match_found = 0;
fp = fopen(filename, "r");
if (fp == NULL) {
printf("Error: could not open file %s\n", filename);
exit(1);
}
while (fgets(line, MAX_LINE_LENGTH, fp) != NULL) {
if (strstr(line, pattern) != NULL) {
printf("%s:%d:%s", filename, line_number, line);
match_found = 1;
}
line_number++;
}
if (!match_found) {
printf("No matches found for %s in %s\n", pattern, filename);
}
fclose(fp);
return 0;
}
Conclusion
This implementation takes two arguments: the pattern to search for and the filename to search in. It then opens the file and reads it line by line using fgets(). For each line, it checks if the pattern appears in the line using strstr(). If a match is found, it prints the filename, line number, and the line containing the match. Finally, if no match is found, it prints a message indicating that no matches were found.
Further Reading:
For further information and examples, Please visit[ C-Programming From Scratch to Advanced 2023-2024]
Top Resources
- Learn-C.org
- C Programming at LearnCpp.com
- GeeksforGeeks - C Programming Language
- C Programming on Tutorialspoint
- Codecademy - Learn C
- CProgramming.com
- C Programming Wikibook
- C Programming - Reddit Community
- C Programming Language - Official Documentation
- GitHub - Awesome C
- HackerRank - C Language
- LeetCode - C