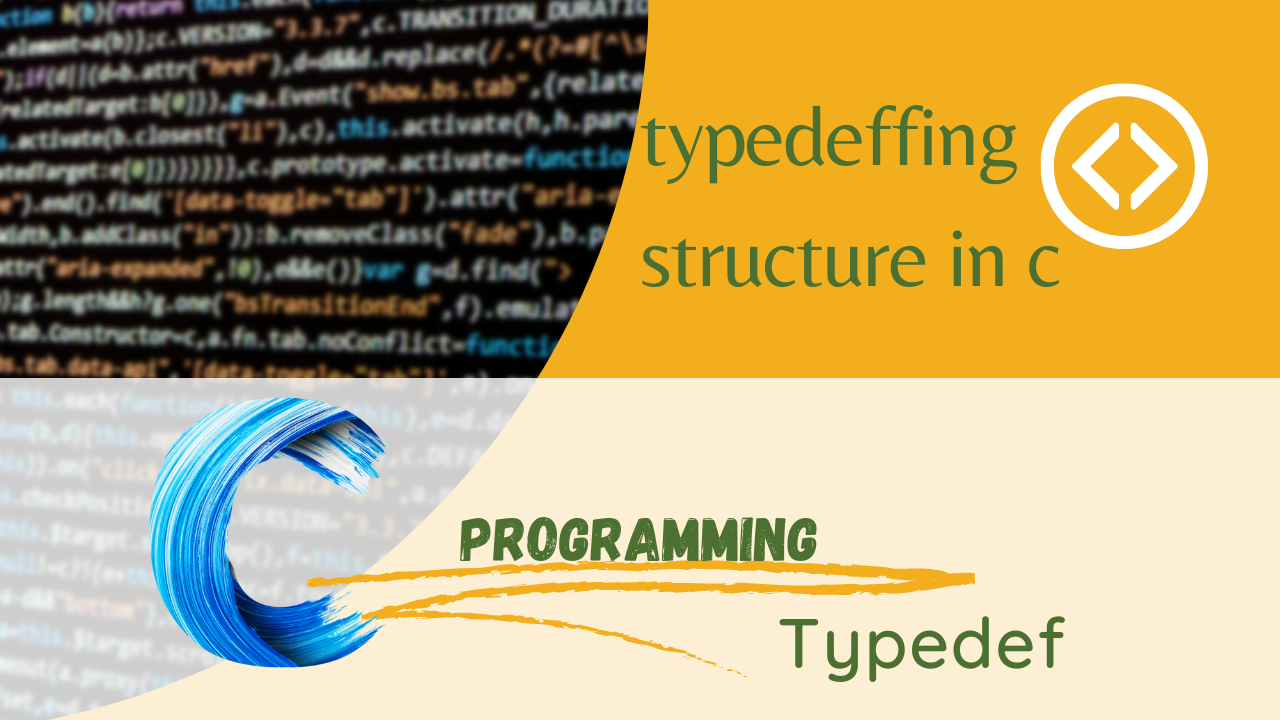
typedeffing a structure in c
Typedeffing a structure in C Programming
To typedef a structure in C, you use the following syntax:
typedef struct {
// Structure members
} struct_name;
For example, the following code defines a structure to represent a student:
typedef struct {
char name[50];
int age;
} Student;
Once you have typedefed a structure, you can use the struct_name identifier to declare variables of that type. For example, the following code declares a variable of type Student:
Student student1;
You can then access the members of the structure using the dot operator (.). For example, the following code accesses the name member of the student1 variable:
printf("%s\n", student1.name);
Benefits of typedeffing structures
There are several benefits to typedeffing structures in C:
- It makes your code more readable and maintainable.
- It reduces the amount of code you need to write.
- It allows you to use the struct_name identifier to declare variables of that type, which can make your code more concise and easier to read.
Example
The following code shows an example of how to use a typedef to define a structure and declare variables of that type:
#include <stdio.h>
typedef struct {
char name[50];
int age;
} Student;
int main() {
Student student1;
printf("Enter the student's name: ");
scanf("%s", student1.name);
printf("Enter the student's age: ");
scanf("%d", &student1.age);
printf("The student's name is %s and their age is %d.\n", student1.name, student1.age);
return 0;
}
Output:
Enter the student's name: Alice
Enter the student's age: 20
The student's name is Alice and their age is 20.
Top Resources
Typedef in c
Typedeffing an array in c
Typedefing unsigned character in c
typedeffing a structure in c
Mastering Typedeffing with Pointers in C Programming
How can typedef be to define a type of structure in c?
What is typedef? What are the advantages and Disadvantages?
What is the difference between #define and typedef in c?
Assignment of Typedef
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!