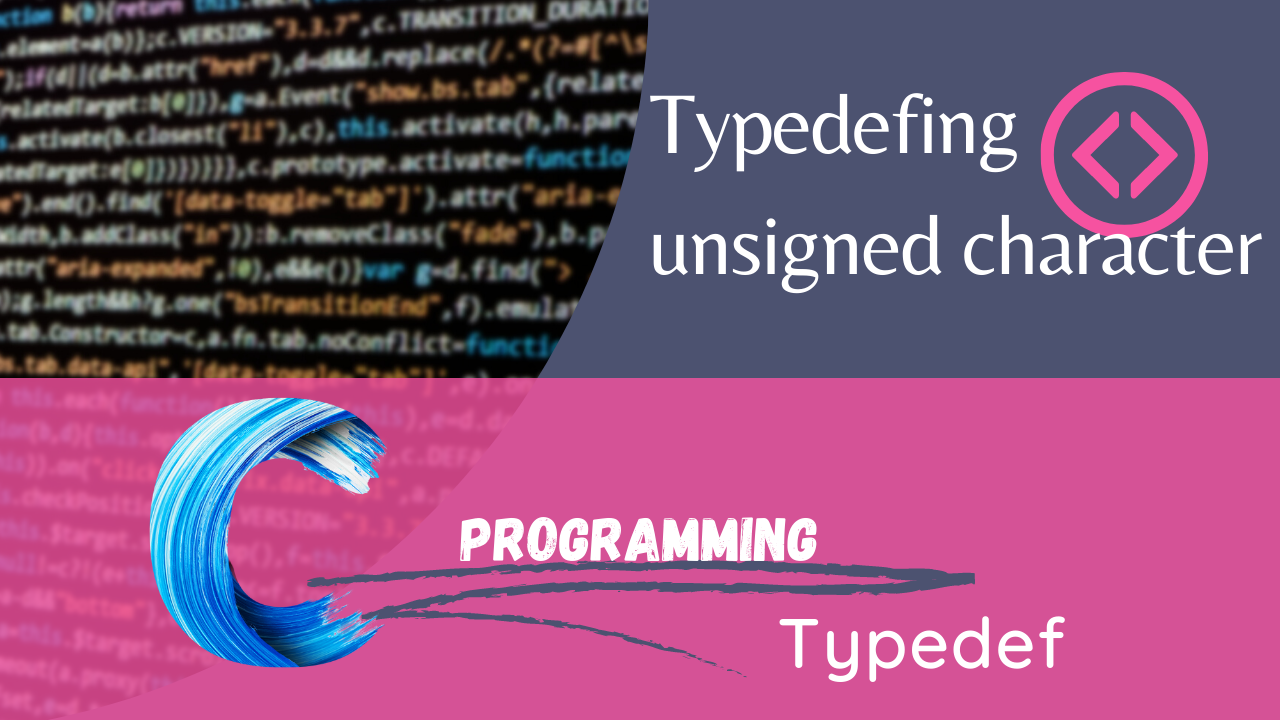
Typedefing unsigned character in c
C Typedef: Creating Aliases for Unsigned Characters
Typedefing an unsigned character in C is a way to create a new data type that is equivalent to the unsigned character data type. This can be useful for making code more readable and maintainable.
To typedef an unsigned character, you use the typedef keyword followed by the new data type name and the existing data type name. For example, the following code typedefs an unsigned character as BYTE:
To typedef an unsigned character in C, you can use the following syntax:
typedef unsigned char BYTE;
This will create a new type called BYTE that is equivalent to an unsigned character. You can then use the BYTE type in your code just like any other type.
For example, the following code shows how to declare and initialize a BYTE variable:
BYTE b = 100;
This code will initialize the variable b with the value 100.
You can also use the typedef keyword to create more complex types. For example, the following code shows how to typedef a type called COLOR that represents a color in RGB:
typedef struct {
BYTE r;
BYTE g;
BYTE b;
} COLOR;
This code creates a new type called COLOR that is a struct with three fields: r, g, and b. Each field is of type BYTE, which means that it can store a value from 0 to 255.
You can then use the COLOR type to declare and initialize variables that represent colors. For example, the following code shows how to declare and initialize a COLOR variable that represents the color red:
COLOR red = {255, 0, 0};
This code will initialize the variable red with the color red.
Using typedefs can make your code more readable and maintainable. It can also help to prevent errors, such as typos.
Here are some examples of how to use typedefs in C:
To typedef a pointer to a character:
typedef char* STRING;
To typedef a struct that represents a point in 2D space:
typedef struct {
float x;
float y;
} POINT;
To typedef an enumeration that represents the days of the week:
typedef enum {
SUNDAY,
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY
} DAY_OF_WEEK;
You can use typedefs anywhere in your code where you would use a regular type. For example, you can use them to declare variables, function parameters, and function return types.
Top Resources
Typedef in c
Typedeffing an array in c
Typedefing unsigned character in c
typedeffing a structure in c
Mastering Typedeffing with Pointers in C Programming
How can typedef be to define a type of structure in c?
What is typedef? What are the advantages and Disadvantages?
What is the difference between #define and typedef in c?
Assignment of Typedef
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!