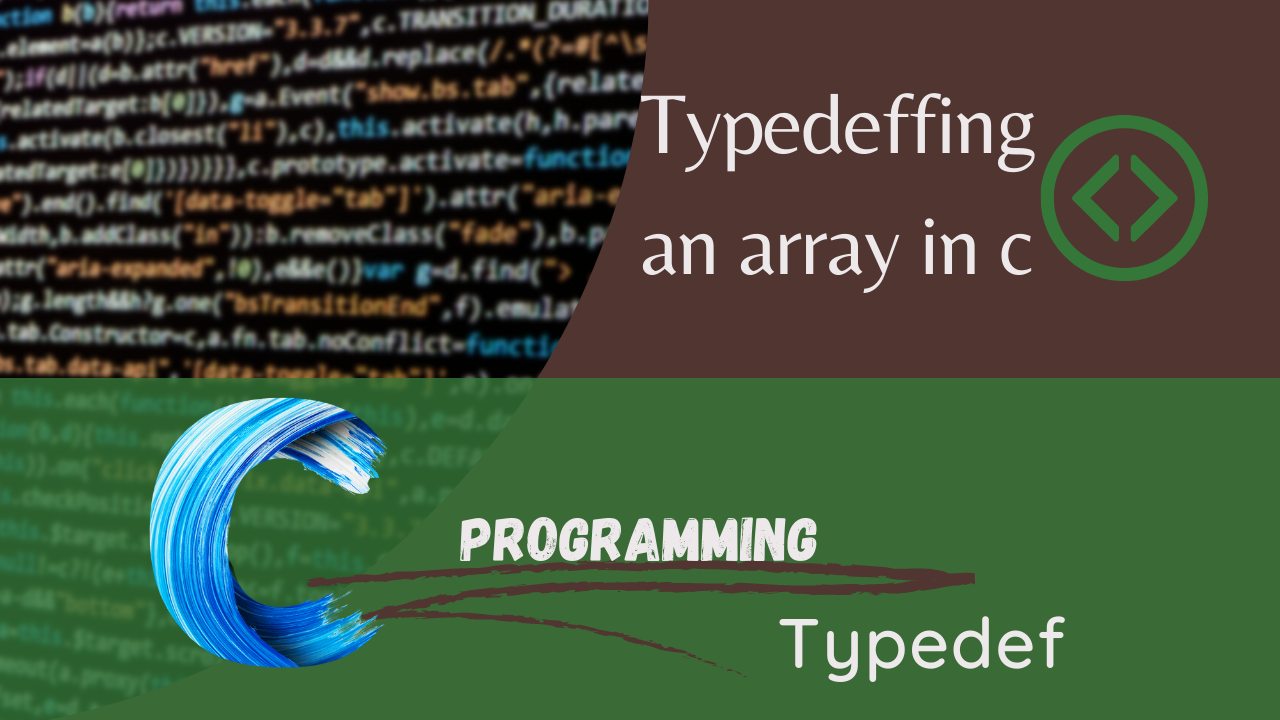
Typedeffing an array in c
Typedef in C: Creating Aliases for Arrays
Typedefing an array in C is a way to create a new data type that is an alias for an existing data type. This can be useful for making code more readable and reusable.
To typedef an array, you use the following syntax:
typedef [];
For example, the following code creates a new data type called IntArray that is an alias for an array of integers:
typedef int IntArray[];
Once you have typedefed an array, you can use the new data type in your code as if it were a built-in data type. For example, the following code declares a variable of type IntArray and initializes it with an array of 5 integers:
IntArray arr = {1, 2, 3, 4, 5};
Typedefing arrays can also be used to pass arrays to functions. For example, the following function takes an array of integers as a parameter:
void printArray(IntArray arr, int size) {
for (int i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
To call this function, you can pass it an array of integers of any size:
IntArray arr = {1, 2, 3, 4, 5};
printArray(arr, 5);
Typedefing arrays is a powerful feature of C that can make your code more readable and reusable.
Some additional benefits of typedefing arrays in C:
- It can make your code more self-documenting.
- It can help you to avoid errors by making it clear what types of data your variables and parameters can hold.
- It can make your code more portable by making it less dependent on the specific sizes of arrays.
Overall, typedefing arrays is a good practice that can make your C code more readable, reusable, and error-free.
Top Resources
Typedef in c
Typedeffing an array in c
Typedefing unsigned character in c
typedeffing a structure in c
Mastering Typedeffing with Pointers in C Programming
How can typedef be to define a type of structure in c?
What is typedef? What are the advantages and Disadvantages?
What is the difference between #define and typedef in c?
Assignment of Typedef
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!