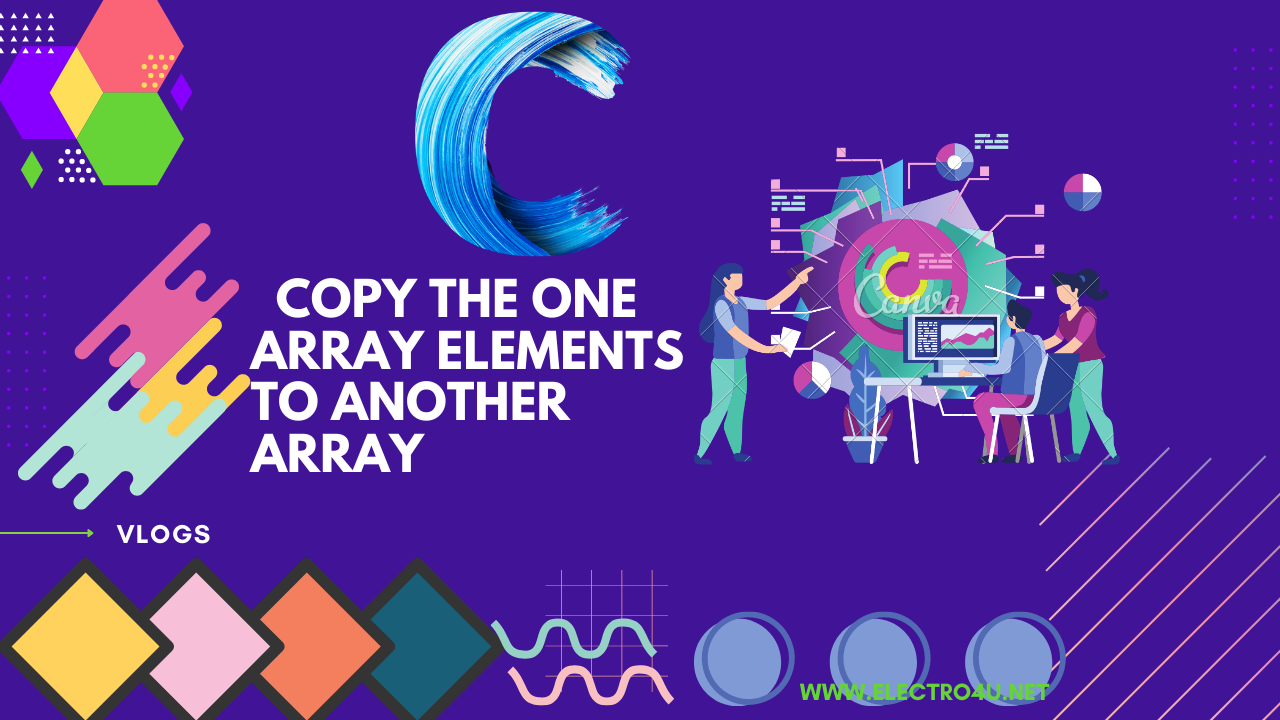
Copy the one array elements to another array in c
Copying Array Elements in C: A Comprehensive Guide
To copy the elements of one array to another array in C, we can use the following steps:
- Declare two arrays of the same type and size.
- Iterate over the first array and copy each element to the second array.
- After the loop has finished iterating, the second array will contain a copy of the first array.
How to copy the elements of one array to another array in C:
C programming
#include <stdio.h>
int main() {
int arr1[] = {1, 2, 3, 4, 5};
int arr2[5];
for (int i = 0; i < 5; i++) {
arr2[i] = arr1[i];
}
// Print the contents of the second array.
for (int i = 0; i < 5; i++) {
printf("%d ", arr2[i]);
}
printf("\n");
return 0;
}
Output:
1 2 3 4 5
We can also use the memcpy() function to copy the elements of one array to another array in C. The memcpy() function copies a block of memory from one location to another.
The function takes three arguments:
- The destination pointer.
- The source pointer.
- The number of bytes to copy.
copy the elements of one array to another array using the memcpy() function,
C programming
#include <stdio.h>
#include <string.h>
int main() {
int arr1[] = {1, 2, 3, 4, 5};
int arr2[5];
// Copy the elements of arr1 to arr2 using the memcpy() function.
memcpy(arr2, arr1, sizeof(arr1));
// Print the contents of the second array.
for (int i = 0; i < 5; i++) {
printf("%d ", arr2[i]);
}
printf("\n");
return 0;
}
Output:
1 2 3 4 5
Which method to use to copy the elements of one array to another array in C depends on your specific needs. If you need to copy a small number of elements, then you can use the for loop method. If you need to copy a large number of elements, then you can use the memcpy()
function.
Top Resources
write a program to copy one array element to another array element in reverse manner.
Write a one-line code to copy the string into the destination.
Copy the one array elements to another array in c
Write a program to copy the source string into the destination string in c
Design a function to copy to copy string into destination string using strcpy and strncpy
Design a recursive function to copy the data source string to destination string
Write a c program to copy data one structure to another structure
Implementation of your own copy command in c
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!