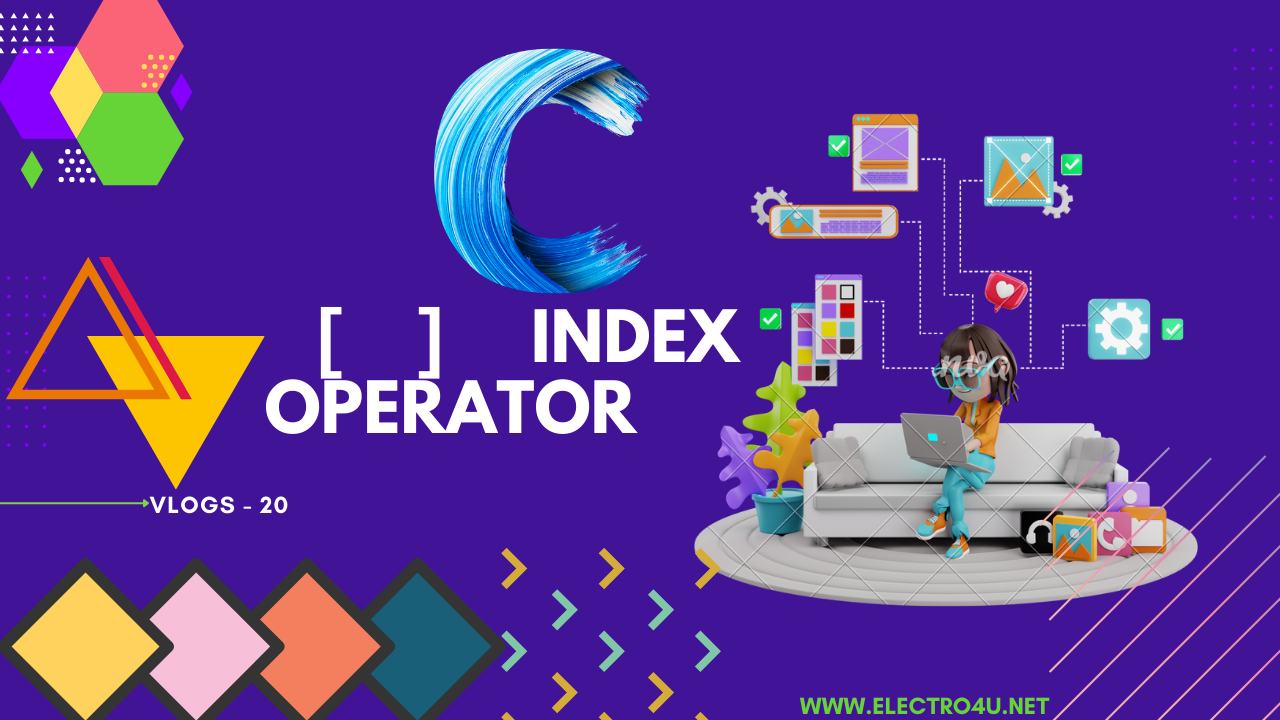
index Operator in c programming
The index operator ([ ])
in C programming is used to access the elements of an array. The index operator takes two operands: the name of the array and the index of the element to be accessed. The index must be an integer expression.
Syntax for the index operator is as follows:
array_name[index]
The array_name is the name of the array and the index is the index of the element to be accessed.
How to use the index operator to access the elements of an array:
int arr[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// Access the first element of the array
int first_element = arr[0];
// Access the last element of the array
int last_element = arr[9];
// Access the element at index 5
int element_at_5 = arr[5];
The index operator can also be used to assign a value to an element of an array.
How to assign the value 100 to the first element of the arr array:
arr[0] = 100;
The index operator can be used with any type of array, including one-dimensional arrays, multidimensional arrays, and arrays of structures.
Some important things to keep in mind when using the index operator:
- The index must be an integer expression.
- The index must be within the bounds of the array. Otherwise, the program will crash.
- The index operator can be used to access and assign values to the elements of an array.
The index operator is a powerful tool for accessing and manipulating arrays in C programming.
Top Resources
Arithmetic operator in c
Assignment operator in c programming
Relational operator in c programming
Logical Operator in c programming
Bitwise operator in c programming
sizeof operator in c programming
Understanding the Comma Operator in C Programming
Dot Operator in c programming
Using && Logical AND Operator in C Programming
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!