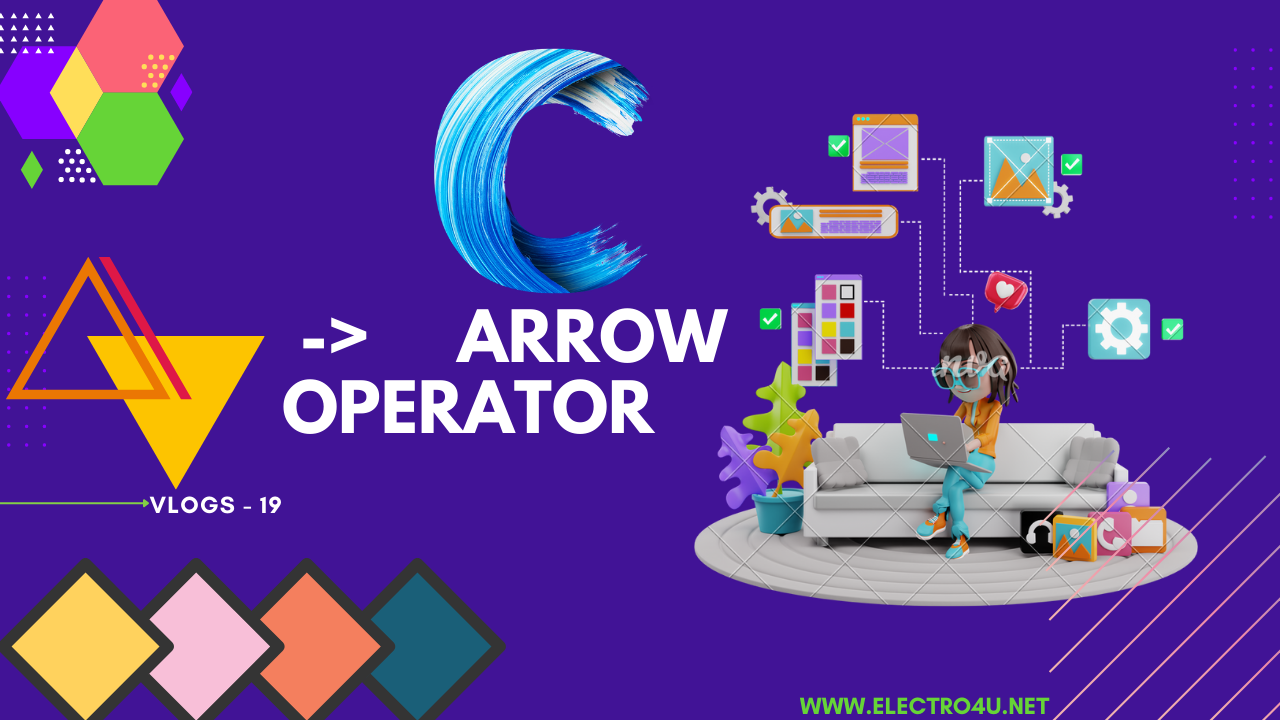
Arrow Operator in c programming
Understanding the Arrow Operator in C Programming
The arrow operator (->)
in C programming is used to access the members of a structure or union using a pointer. It is a shorthand for the dot operator (.)
when used with pointers.
The arrow operator is more efficient than the dot operator when used with pointers, as it avoids the need to dereference the pointer twice.
syntax for the arrow operator is as follows:
pointer->member
where pointer is a pointer to a structure or union, and member is a member of the structure or union.
How to use the arrow operator to access the members of a structure:
struct Student {
int age;
char name[10];
};
int main() {
struct Student student;
student.age = 20;
strcpy(student.name, "John Doe");
struct Student* student_ptr = &student;
printf("The student's name is %s and their age is %d\n", student_ptr->name, student_ptr->age);
return 0;
}
Output:
The student's name is John Doe and their age is 20
The arrow operator can also be used to access the members of a union. The syntax is the same as for accessing the members of a structure.
The arrow operator can be a useful tool for writing concise and efficient code. However, it is important to use it carefully, as it can make code more difficult to read and debug.
Some tips for using the arrow operator:
- Only use the arrow operator with pointers to structures and unions.
- Be careful when using the arrow operator to access members of a structure or union that is not initialized.
- Avoid using the arrow operator to access members of a structure or union that is NULL.
Top Resources
Arithmetic operator in c
Assignment operator in c programming
Relational operator in c programming
Logical Operator in c programming
Bitwise operator in c programming
sizeof operator in c programming
Understanding the Comma Operator in C Programming
Dot Operator in c programming
Using && Logical AND Operator in C Programming
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!