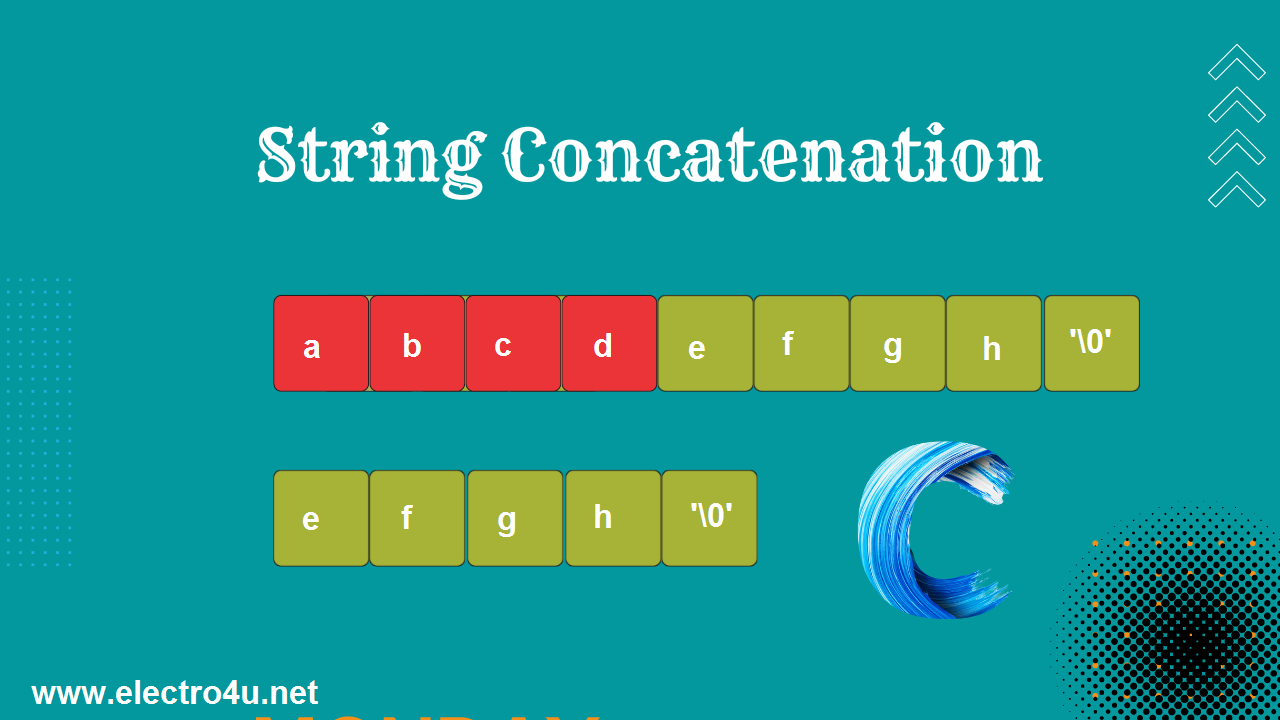
Write a program to concatenate two string in c
C program to concatenate two strings
Concatenating two strings means joining them together to form a single string. In C, string concatenation is performed using the strcat function, which is available in the standard C library.
Introduction
This program demonstrates how to concatenate two strings in C. Concatenation is the process of joining two strings together to form a new string. This is a common operation in C programming, and it is used in many different applications, such as text processing, data manipulation, and web development.
How to use the strcat() function to concatenate two strings:
#include <stdio.h>
#include <string.h>
int main() {
char str1[100], str2[100], concatenatedStr[200];
printf("Enter the first string: ");
scanf("%s", str1);
printf("Enter the second string: ");
scanf("%s", str2);
// Concatenate the two strings into the concatenatedStr variable
strcat(concatenatedStr, str1);
strcat(concatenatedStr, str2);
// Print the concatenated string
printf("The concatenated string is: %s\n", concatenatedStr);
return 0;
}
Output
Enter the first string: Hello
Enter the second string: World
The concatenated string is: HelloWorld
Conclusion
This program demonstrates how to concatenate two strings in C. The strcat() function is a simple and efficient way to join two strings together to form a new string.
Further Reading:
Concatenating Two Strings in C: A Step-by-Step Guide
Design a function Concatenate two string in c
program to concatenate two strings without using strcat() function.
Design a function to count given character in given string
Design a function to reverse the given string
Design a function to search given character in given string in c
Design a function to reverse the word in given string
Design a function replace the words in reverse order in a given string line
Design a function to find string length in c
Design a Function to print the string character by character
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [[email protected]].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!