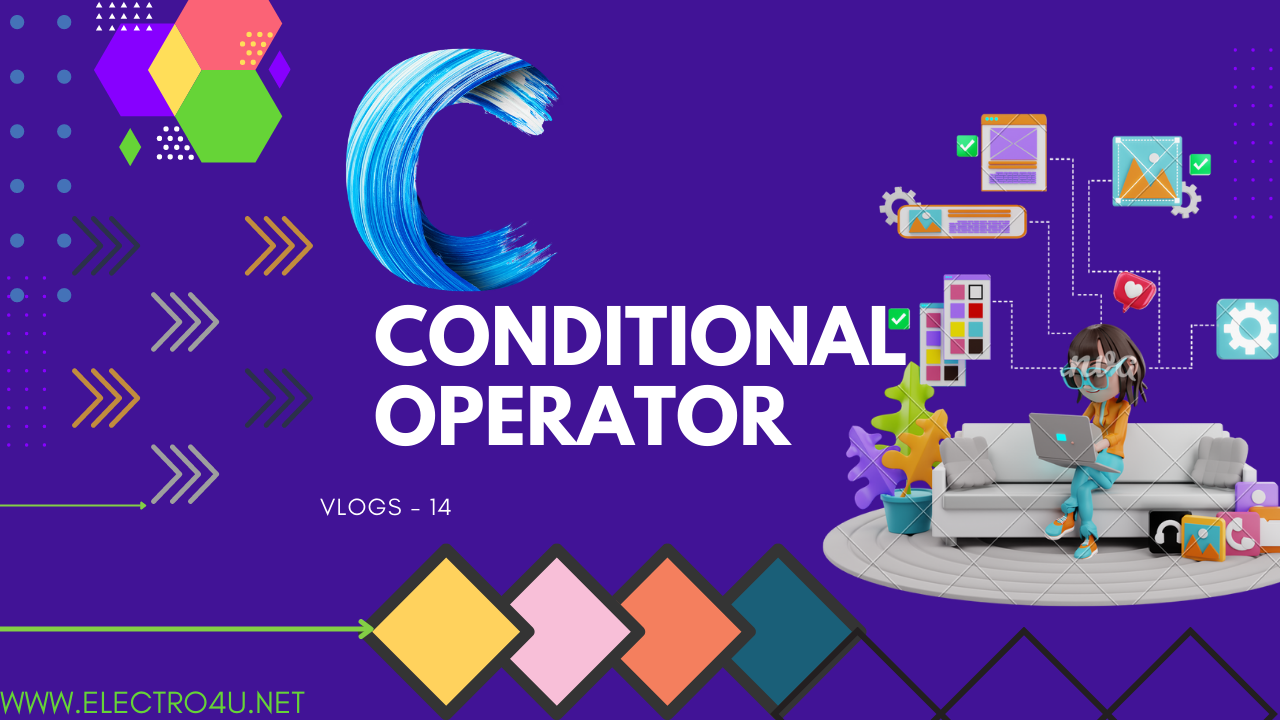
conditional operator in c
C Conditional Operator (?:) Explained | Ternary Operator in C
The conditional operator in C, also known as the ternary operator, is a shorthand for writing an if-else statement. It takes three operands: a condition, an expression to be evaluated if the condition is true, and an expression to be evaluated if the condition is false. The result of the conditional operator is the value of the expression that is evaluated.
Syntax
condition ? expression_if_true : expression_if_false;
The condition must be an expression that evaluates to a Boolean value. The expression_if_true and expression_if_false expressions can be any valid C expression.
Code shows how to use the conditional operator to write a simple if-else statement:
int x = 10;
int y = 20;
int max = x > y ? x : y;
printf("The maximum of %d and %d is %d\n", x, y, max);
This code will print the following output:
The maximum of 10 and 20 is 20
The following code shows how to use the conditional operator to assign a value to a variable:
int x = 10;
int y = 20;
int max = x > y ? x : y;
int min = x < y ? x : y;
printf("The maximum of %d and %d is %d\n", x, y, max);
printf("The minimum of %d and %d is %d\n", x, y, min);
This code will print the following output:
The maximum of 10 and 20 is 20
The minimum of 10 and 20 is 10
The conditional operator can be used in any place where an expression is valid. For example, it can be used in function arguments, function returns, and loop conditions.
The conditional operator can be a useful tool for writing concise and efficient code. However, it is important to use it carefully, as it can make code more difficult to read and debug.
Top Resources
Arithmetic operator in c
Assignment operator in c programming
Relational operator in c programming
Logical Operator in c programming
Bitwise operator in c programming
sizeof operator in c programming
Understanding the Comma Operator in C Programming
Dot Operator in c programming
Using && Logical AND Operator in C Programming
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!