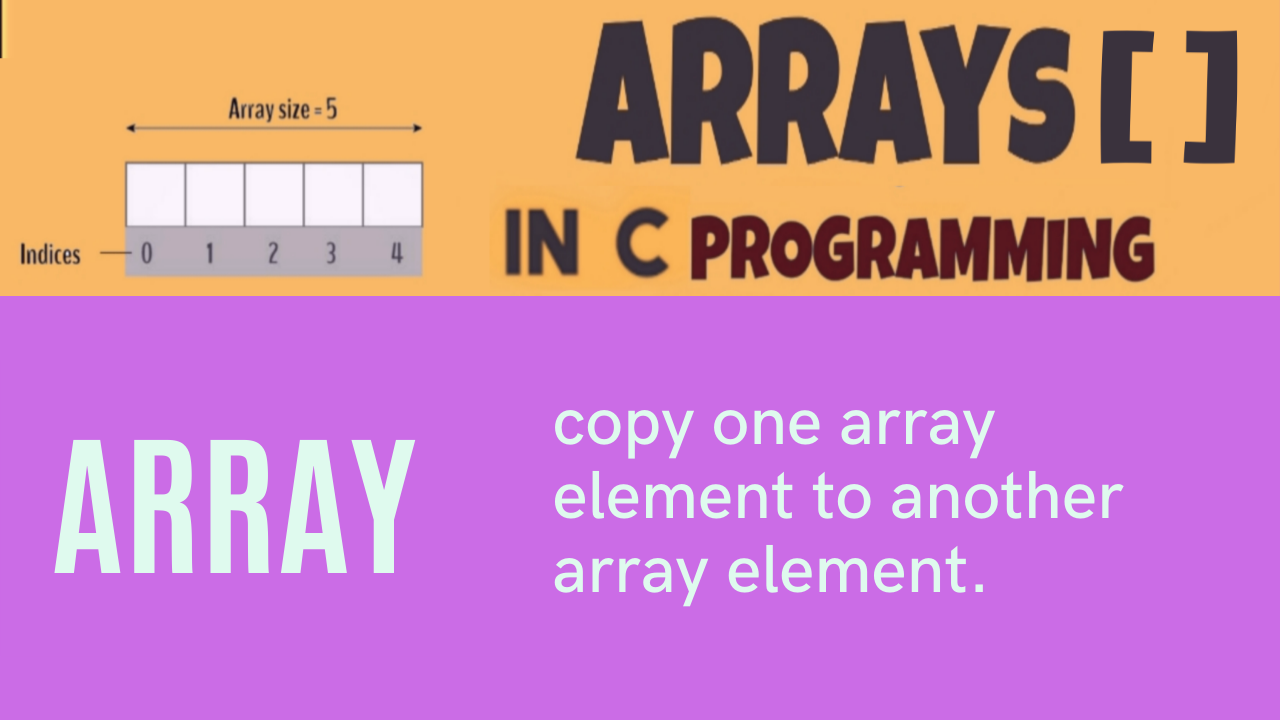
write a program to copy one array element to another array element.
Copy Elements from One Array to Another in C
Writing a C program to copy one array element to another array element means creating a program in the C programming language that takes elements from one array and copies them to another array. This can be useful in many different programming applications, such as when you want to manipulate data in one array without altering the original data.
The program typically involves declaring two arrays of the same type and size, iterating through the first array and copying each element to the corresponding index in the second array. The copied elements should have the same data type and value as the original elements. Once the copying is complete, the program may then print out the elements of the second array to confirm that they have been copied correctly.
C to copy elements from one array to another:
#include
int main() {
int source[] = {1, 2, 3, 4, 5};
int destination[5];
int n = sizeof(source)/sizeof(source[0]);
// Copy elements from source to destination array
for (int i = 0; i < n; i++) {
destination[i] = source[i];
}
// Print the elements of the destination array
printf("Destination Array:\n");
for (int i = 0; i < n; i++) {
printf("%d ", destination[i]);
}
return 0;
}
Output of the C program you provided is:
Destination Array:
1 2 3 4 5
In this program, we declare two integer arrays: source
and destination,
both of size n. We then iterate through the source array and copy its elements to the corresponding indices in the destination
array using a for
loop. Finally, we print the elements of the destination
array using another for
loop.
This program demonstrates how to copy elements from one array to another in C, which can be useful in many different programming applications.
Further Reading:
write a program to find the largest elements of a given array with an index.
write a c program to find the second largest elements of a given array.
write a program to find the first largest and second-largest elements of a given array.
Write a c program find largest elements in this array number or index
Write a C program to find out the second largest and second smallest elements of any unsorted array without using any shorting Technique
write a program to find the smallest elements of a given array with an index.
write a program to find the first largest and first smallest elements of a given array.
write a program to find the second smallest elements of a given array.
write a program to find the second largest and second smallest elements of a given array.
write a program to find the first smallest and second smallest elements of a given array.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.